Stochastic Simulation concepts in Python are worked effectively by our technical experts we help you to design and evaluate models which are chance-based or basically indefinite, stochastic simulation often engages in development of random variables. As a means to carry out stochastic simulations in a simpler manner, Python provides numerous libraries together with scipy and numpy. We recommend an instance of a basic stochastic simulation with the aid of Python to design a random walk:
Instance: Random Walk Simulation
A sequence of random steps is encompassed in a random walk which is examined as a mathematical solution of a path. The following instance will simulate a basic one-dimensional random walk.
Step 1: Install Required Libraries
Initially, it is advisable to assure that we have installed matplotlib and numpy for visualization and numerical processes:
pip install numpy matplotlib
Step 2: Write the Simulation Code
import numpy as np
import matplotlib.pyplot as plt
# Parameters
n_steps = 1000 # Number of steps in the random walk
start_position = 0 # Starting position of the walk
# Generate random steps
steps = np.random.choice([-1, 1], size=n_steps) # Randomly choose -1 or 1 for each step
positions = np.cumsum(steps) # Cumulative sum to get the positions
# Include the starting position
positions = np.insert(positions, 0, start_position)
# Plot the random walk
plt.figure(figsize=(10, 6))
plt.plot(positions, label=”Random Walk”)
plt.xlabel(“Step”)
plt.ylabel(“Position”)
plt.title(“1D Random Walk”)
plt.legend()
plt.grid(True)
plt.show()
Step 3: Explanation of the Code
- Imports: Generally, the essential libraries should be imported such as matplotlib for plotting and numpy for numerical processes.
- Parameters: Focus on describing the initial position and number of steps.
- Random Steps: An array of random steps has to be produced in which every step is defined as either 1 or -1.
- Cumulative Sum: As a means to obtain the position at every step, it is beneficial to employ the cumulative sum (np.cumsum).
- Insert Start Position: At the origin of the positions array, our team aims to add the initial position.
- Plotting: To plot the positions of the random walk, we employ matplotlib.
Advanced Instance: Stochastic Process (Geometric Brownian Motion)
To design stock prices in finance, Geometric Brownian Motion (GBM) is employed which is a continuous-time stochastic procedure. The following is an instance of simulating GBM:
Step 1: Write the Simulation Code
import numpy as np
import matplotlib.pyplot as plt
# Parameters
S0 = 100 # Initial stock price
mu = 0.1 # Drift coefficient
sigma = 0.2 # Volatility coefficient
T = 1.0 # Time period (1 year)
N = 1000 # Number of time steps
dt = T / N # Time step size
# Time array
t = np.linspace(0, T, N+1)
# Generate random variables for the Brownian motion
dW = np.random.normal(0, np.sqrt(dt), size=N)
W = np.cumsum(dW) # Cumulative sum to simulate the Brownian motion
# Simulate the GBM
S = S0 * np.exp((mu – 0.5 * sigma**2) * t[1:] + sigma * W)
S = np.insert(S, 0, S0) # Include the initial stock price
# Plot the GBM
plt.figure(figsize=(10, 6))
plt.plot(t, S, label=”Geometric Brownian Motion”)
plt.xlabel(“Time”)
plt.ylabel(“Stock Price”)
plt.title(“Geometric Brownian Motion Simulation”)
plt.legend()
plt.grid(True)
plt.show()
Step 2: Explanation of the Code
- Parameters: Typically, parameters such as initial stock price (S0), volatility coefficient (sigma), number of time steps (N), drift coefficient (mu), and total time period (T) ought to be described.
- Time Array: With N+1 points, we intend to develop a time array from 0 to T.
- Brownian Motion: For the extensions of the Brownian motion, it is significant to produce random usual variables. To simulate the Brownian path, we plan to calculate the cumulative sum.
- GBM Simulation: In order to simulate the stock price path, our team focuses on utilizing the formula for GBM.
- Plotting: For plotting the simulated stock price path, it is advisable to utilize matplotlib.
Additional Reading and Libraries
- numpy: It is significant to acquire the benefit of numPy to conduct numerical processes and produce random numbers.
- stats: Generally, for statistical distributions and operations, it is considered as beneficial.
- matplotlib: This library is useful for plotting and visualization.
- pandas: Mainly, pandas are valuable for financial simulations as it manages time series data in an effective manner.
stochastic simulation python projects
In the contemporary years, several stochastic simulation projects are emerging continuously. Encompassing different domains and uses, an extensive collection of 100 stochastic simulation projects in Python are offered by us:
Basic Simulations
- 2D Random Walk Simulation
- Coin Toss Simulation
- Monte Carlo Integration
- Simulating Pi with Monte Carlo Method
- Simulating Normal Distribution
- Simulating Geometric Brownian Motion
- Simulating Brownian Motion
- Simulating Bernoulli Trials
- Simulating Uniform Distribution
- Simulating Beta Distribution
- 1D Random Walk Simulation
- 3D Random Walk Simulation
- Dice Roll Simulation
- Buffon’s Needle Problem
- Simulating Exponential Distribution
- Simulating Poisson Process
- Simulating Wiener Process
- Simulating Markov Chains
- Simulating Binomial Distribution
- Simulating Gamma Distribution
Finance
- Option Pricing with Monte Carlo Simulation
- Risk Management Simulation
- Simulating Credit Risk
- Value at Risk (VaR) Simulation
- Simulating Black-Scholes Model
- Stock Price Simulation with Geometric Brownian Motion
- Portfolio Optimization with Monte Carlo Simulation
- Simulating Interest Rate Models
- Simulating Default Risk
- Stress Testing Financial Models
Biology and Medicine
- Simulating Epidemic Spread (SIR Model)
- Simulating Predator-Prey Dynamics (Lotka-Volterra)
- Simulating Mutation Rates
- Simulating Tumor Growth
- Simulating Evolutionary Algorithms
- Simulating Population Growth (Logistic Model)
- Genetic Drift Simulation
- Simulating Cell Division
- Simulating Drug Dosage and Effectiveness
- Simulating Enzyme Kinetics
Engineering and Physics
- Simulating Particle Movement in Fluids
- Simulating Solar Energy Production
- Simulating Nuclear Decay
- Simulating Traffic Flow
- Simulating Mechanical Systems with Random Forces
- Simulating Heat Diffusion
- Simulating Electrical Circuits with Noise
- Simulating Wind Turbine Performance
- Simulating Projectile Motion with Air Resistance
- Simulating Renewable Energy Systems
Computer Science
- Simulating Queue Systems
- Simulating Algorithm Performance with Random Inputs
- Simulating Social Networks
- Simulating Fault Tolerance in Systems
- Simulating Data Transmission Errors
- Simulating Network Traffic
- Simulating Load Balancing in Distributed Systems
- Simulating Random Graphs (Erdős–Rényi Model)
- Simulating Search Algorithms on Random Data
- Simulating Cybersecurity Attacks
Economics and Social Sciences
- Simulating Consumer Behavior
- Simulating Voting Systems
- Simulating Game Theory Models
- Simulating Urban Development
- Simulating Resource Allocation
- Simulating Market Dynamics
- Simulating Auction Models
- Simulating Economic Growth Models
- Simulating Social Mobility
- Simulating Income Distribution
Environmental Science
- Simulating Forest Fire Spread
- Simulating Pollution Dispersion
- Simulating Ecosystem Dynamics
- Simulating Natural Disaster Impact
- Simulating Carbon Emissions
- Simulating Climate Change Models
- Simulating Water Resource Management
- Simulating Wildlife Migration Patterns
- Simulating Soil Erosion
- Simulating Renewable Energy Integration
Manufacturing and Operations
- Simulating Inventory Management
- Simulating Quality Control Processes
- Simulating Manufacturing Yield
- Simulating Logistics and Distribution
- Simulating Lean Manufacturing Processes
- Simulating Production Lines
- Simulating Supply Chain Dynamics
- Simulating Maintenance Schedules
- Simulating Assembly Line Efficiency
- Simulating Workforce Management
Miscellaneous
- Simulating Sports Events
- Simulating Voting Poll Predictions
- Simulating Music Popularity Trends
- Simulating Urban Traffic Patterns
- Simulating Online Marketplaces
- Simulating Board Games
- Simulating Gambling Games
- Simulating Art Auction Prices
- Simulating Language Evolution
- Simulating Customer Service Queues
Through this article, we have provided a basic stochastic simulation with Python to design a random walk. Also, a thorough set of 100 stochastic simulation projects in Python involving numerous disciplines and uses are suggested by us in an explicit manner.
Get innovative Stochastic Simulation concepts in Python from our team of technical experts. We cover a wide range of areas, offering you the finest project ideas and topics. Share your requirements with us, and we will assist you in achieving optimal simulation outcomes.
Subscribe Our Youtube Channel
You can Watch all Subjects Matlab & Simulink latest Innovative Project Results
Our services
We want to support Uncompromise Matlab service for all your Requirements Our Reseachers and Technical team keep update the technology for all subjects ,We assure We Meet out Your Needs.
Our Services
- Matlab Research Paper Help
- Matlab assignment help
- Matlab Project Help
- Matlab Homework Help
- Simulink assignment help
- Simulink Project Help
- Simulink Homework Help
- Matlab Research Paper Help
- NS3 Research Paper Help
- Omnet++ Research Paper Help
Our Benefits
- Customised Matlab Assignments
- Global Assignment Knowledge
- Best Assignment Writers
- Certified Matlab Trainers
- Experienced Matlab Developers
- Over 400k+ Satisfied Students
- Ontime support
- Best Price Guarantee
- Plagiarism Free Work
- Correct Citations
Expert Matlab services just 1-click
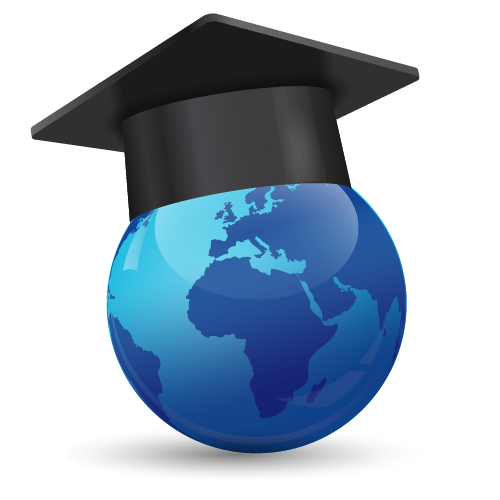
Delivery Materials
Unlimited support we offer you
For better understanding purpose we provide following Materials for all Kind of Research & Assignment & Homework service.
Programs
Designs
Simulations
Results
Graphs
Result snapshot
Video Tutorial
Instructions Profile
Sofware Install Guide
Execution Guidance
Explanations
Implement Plan
Matlab Projects
Matlab projects innovators has laid our steps in all dimension related to math works.Our concern support matlab projects for more than 10 years.Many Research scholars are benefited by our matlab projects service.We are trusted institution who supplies matlab projects for many universities and colleges.
Reasons to choose Matlab Projects .org???
Our Service are widely utilized by Research centers.More than 5000+ Projects & Thesis has been provided by us to Students & Research Scholars. All current mathworks software versions are being updated by us.
Our concern has provided the required solution for all the above mention technical problems required by clients with best Customer Support.
- Novel Idea
- Ontime Delivery
- Best Prices
- Unique Work
Simulation Projects Workflow

Embedded Projects Workflow
