Simulated Annealing MATLAB is an important as well as challenging process that has to be conducted by adhering to several guidelines. We have specified work format so that you get your work done at high quality within the specified time frame. In order to execute this in MATLAB, we list out the fundamental procedures in an explicit and brief manner:
Fundamental Procedures for Simulated Annealing Implementation:
- Specify the Objective Function:
- Initially, the major objective function f(x)f(x)f(x) which we aim to increase or decrease has to be specified. This function must output a scalar value by considering the existing condition xxx as input. The objective function’s assessment at xxx is depicted in the scalar value.
- Set Parameters:
- Preliminary parameters have to be described. It could include initial solution xinitialx_{\text{initial}}xinitial, initial temperature TinitialT_{\text{initial}}Tinitial, highest iterations (or final state), and cooling schedule.
- Create Initial Solution:
- By utilizing a heuristic approach or in a random manner, we have to produce a preliminary solution xcurrentx_{\text{current}}xcurrent.
- Simulated Annealing Iteration:
- Till a final state is reached, the process must be iterated (for instance: highest iterations attained).
- Produce a Neighbor Solution:
- In order to produce a neighboring solution xnewx_{\text{new}}xnew, the existing solution xcurrentx_{\text{current}}xcurrent has to be perturbed. Through any appropriate perturbation technique, exchanging components, or flipping bits, this process can be conducted.
- Assess Objective Function:
- Focus on calculating ΔE=f(xnew)−f(xcurrent)\Delta E = f(x_{\text{new}}) – f(x_{\text{current}})ΔE=f(xnew)−f(xcurrent).
- Acceptance Probability:
- The acceptance probability P=e−ΔE/TP = e^{-\Delta E / T}P=e−ΔE/T should be estimated. Here, the existing temperature is indicated as TTT.
- Decision Making:
- A random value has to be produced as r∈[0,1]r \in [0, 1]r∈[0,1].
- As the novel existing solution xcurrentx_{\text{current}}xcurrent, take xnewx_{\text{new}}xnew, if r<Pr < Pr<P.
- If the condition is not satisfied, preserve xcurrentx_{\text{current}}xcurrent.
- Cooling Schedule Update:
- Through utilizing a cooling schedule, the temperature TTT must be upgraded (for instance: Tnew=Tcurrent×αT_{\text{new}} = T_{\text{current}} \times \alphaTnew=Tcurrent×α, in which α\alphaα signifies a cooling rate).
- Termination:
- While the final state is reached, we need to stop the iteration (for example: when highest iterations attained, temperature falls under a threshold).
Sample MATLAB Execution:
For a basic optimization issue, a simple execution of simulated annealing is depicted by us through a fundamental MATLAB code snippet:
% Objective function example (Rosenbrock function)
f = @(x) (1-x(1))^2 + 100*(x(2)-x(1)^2)^2;
% Parameters
T_initial = 100; % Initial temperature
alpha = 0.95; % Cooling rate
max_iter = 1000; % Maximum iterations
x_initial = [rand(), rand()]; % Initial solution
% Initialize
x_current = x_initial;
T = T_initial;
% Simulated Annealing loop
for iter = 1:max_iter
% Generate neighbor solution
x_new = x_current + randn(size(x_current))*0.1; % Example perturbation
% Evaluate objective function
E_current = f(x_current);
E_new = f(x_new);
% Calculate delta E
delta_E = E_new – E_current;
% Acceptance probability
if delta_E < 0 || rand() < exp(-delta_E / T)
x_current = x_new; % Accept the new solution
end
% Cooling schedule update
T = T * alpha;
% Display current best solution
disp([‘Iteration ‘, num2str(iter), ‘: Best solution = ‘, num2str(x_current)]);
% Termination condition (e.g., temperature threshold)
if T < 1e-3
break;
end
end
disp(‘Simulated Annealing finished.’);
disp([‘Best solution found: x = ‘, num2str(x_current)]);
disp([‘Objective function value: ‘, num2str(f(x_current))]);
Hints:
- Objective Function: Using the particular objective function, swap f.
- Perturbation: On the basis of the problem field, we have to adapt the neighbor generation approach (x_new).
- Cooling Schedule: To stabilize analysis and manipulation, experiments have to be carried out by utilizing various cooling rates (alpha).
- Termination Condition: In terms of the problem’s necessities, adjust the final state.
Important 50 simulated annealing matlab Project Topics
Across numerous fields, simulated annealing is employed in an extensive manner and is referred to as a probabilistic approach. Related to simulated annealing usage in MATLAB, we recommend 50 important project topics, along with concise explanations:
- Traveling Salesman Problem (TSP):
- With the aim of reducing travel distance, an ideal or near-ideal tour has to be identified for the TSP by applying simulated annealing.
- Job Scheduling:
- To reduce resource usage or finishing time, job planning on several machines must be enhanced with simulated annealing.
- Vehicle Routing Problem (VRP):
- For several vehicles which deliver products to consumers, we improve paths by employing simulated annealing. This plan is specifically for reducing overall time or distance.
- Bin Packing Problem:
- In order to reduce the overall wasted area or amount of bins utilized, pack products into bins in an effective manner through the use of simulated annealing.
- Graph Coloring Problem:
- Using the least amount of colors, color a graph by implementing simulated annealing. Note that the similar color should not be presented in two continuous vertices.
- Knapsack Problem:
- The ideal subset of products has to be identified, which does not surpass the knapsack’s volume but enhances the overall value. For that, we employ simulated annealing.
- Facility Location Problem:
- To reduce the distance or overall cost to consumers, the location of amenities must be improved with the aid of simulated annealing.
- Feature Selection in Machine Learning:
- A subset of characteristics should be chosen, which enhance the machine learning model’s forecasting preciseness. For that, our project utilizes simulated annealing.
- Network Design Optimization:
- As a means to improve strength or reduce expense, an ideal network design (for instance: telecommunications, computer networks) has to be modeled with simulated annealing.
- VLSI Circuit Layout Optimization:
- To reduce overall area or wire length, the deployment and routing of VLSI circuits has to be enhanced on a chip. To accomplish this goal, we make use of simulated annealing.
- Protein Folding Problem:
- The innate conformation of a protein structure must be forecasted, which enhances strength or reduces energy. For that, employ simulated annealing.
- Job Shop Scheduling:
- With the intention of decreasing overall completion duration or makespan in a manufacturing platform, the jobs have to be scheduled by utilizing simulated annealing.
- Portfolio Optimization:
- To improve profits in addition to following conditions or reducing vulnerabilities, investment portfolios should be enhanced with the support of simulated annealing.
- Wireless Sensor Network Deployment:
- In order to reduce energy usage and enhance coverage, we implement wireless sensor nodes by means of simulated annealing.
- Optimal Control of Dynamical Systems:
- Enhance control parameters by regulating dynamical frameworks with simulated annealing. Some of the potential frameworks are chemical processes, robotic arms, and others.
- Sequence Alignment in Bioinformatics:
- Make use of simulated annealing to adapt sequences (for example: protein, DNA). This is majorly for detecting progressive connections and enhancing correspondence rates.
- Image Segmentation:
- To enhance region similarity and boundary identification, the images have to be divided into relevant areas through the use of simulated annealing.
- Optimal Power Flow in Electrical Grids:
- In electrical grids, power generation and distribution must be improved to enhance effectiveness or reduce expenses. To achieve this goal, we utilize simulated annealing.
- Satellite Constellation Design:
- For worldwide coverage, ideal satellite arrangements have to be modeled with simulated annealing. This project plan intends to enhance revisit durations or reduce coverage gaps.
- Resource Allocation in Cloud Computing:
- Aim to improve performance or reduce expenses in cloud platforms by allocating resources (for instance: bandwidth, virtual machines) with simulated annealing.
- Optimal Sensor Placement:
- To reduce overlap and enhance coverage, sensors should be deployed in a platform with the aid of simulated annealing.
- Routing Optimization in Wireless Mesh Networks:
- With the focus on improving throughput or decreasing delays in wireless mesh networks, we enhance routing paths through simulated annealing.
- Video Streaming Optimization:
- Our project aims to improve user contentment or reduce buffering by enhancing video streaming quality (for instance: bitrate adaptation). For that, it employs simulated annealing.
- Dynamic Pricing Optimization:
- As a means to enhance market share or returns, the pricing policies have to be improved in a dynamic manner by means of simulated annealing.
- Natural Language Processing (NLP) Tasks:
- In various NLP missions like machine translation or text summarization, enhance parameters by implementing simulated annealing.
- Healthcare Resource Allocation:
- To improve patient care effectiveness, the healthcare resources (for instance: medical staff, hospital beds) must be allocated with the help of simulated annealing.
- Optimal Urban Planning:
- With the aim of improving inhabitability or reducing congestion, urban structures (for example: residential areas, transportation networks) have to be designed through a simulated annealing approach.
- Vehicle Fleet Management:
- Concentrate on employing simulated annealing to handle vehicle fleets (for instance: delivery vehicles, taxis), specifically for plan and route enhancement.
- Energy Management in Smart Grids:
- In order to reduce ecological implication or expenses in smart grids, we improve energy usage and distribution with the method of simulated annealing.
- Supply Chain Optimization:
- To reduce expenses or enhance effectiveness, supply chain logistics (for instance: transportation, inventory handling) should be improved by means of simulated annealing technique.
- Internet of Things (IoT) Network Optimization:
- For reducing energy usage and improving coverage, enhance the placements of IoT networks by utilizing simulated annealing.
- Multi-objective Optimization:
- As a means to identify Pareto-optimal solutions, multi-objective optimization issues have to be addressed with a simulated annealing technique.
- Automated Parameter Tuning for Algorithms:
- The parameters of machine learning algorithms must be adapted in an automatic manner to enhance performance indicators. For that, we employ simulated annealing.
- Optimal Resource Allocation in Multi-agent Systems:
- Across several agents (for instance: automatic vehicles, robots), resources have to be allocated with simulated annealing. Through this project plan, the entire system performance has to be enhanced.
- Fuzzy System Parameter Optimization:
- To enhance framework strength and preciseness, the parameters of fuzzy logic frameworks should be improved with the aid of simulated annealing technique.
- Dynamic Spectrum Allocation in Cognitive Radio Networks:
- For enhancing spectrum usage in cognitive radio networks, spectrum has to be allocated in a dynamic way by utilizing a simulated annealing method.
- Production Planning and Scheduling:
- In order to reduce interruption and enhance resource allocation in manufacturing, the production processes have to be planned and arranged with the technique of simulated annealing.
- Autonomous Vehicle Path Planning:
- To carry out navigation in an effective and secure manner, ideal routes must be scheduled for self-driving vehicles by means of simulated annealing.
- Agricultural Yield Optimization:
- The agricultural approaches (for example: irrigation planning, crop rotation) should be enhanced through the use of a simulated annealing technique. This project mainly intends to reduce resource utilization and improve production.
- Network Security Parameter Optimization:
- By utilizing the simulated annealing method, the parameters of network security frameworks (for instance: intrusion detection systems) have to be enhanced, especially for threat identification improvement.
- Dynamic Pricing Strategy Optimization:
- As a means to enhance consumer contentment and return in e-commerce, the pricing policies must be improved in a dynamic manner with the approach of simulated annealing.
- Personalized Recommendation Systems:
- On the basis of user choices, the product suggestions should be improved by creating customized recommendation frameworks. For that, we make use of a simulated annealing technique.
- Optimal Resource Allocation in Virtualized Environments:
- To enhance resource usage and performance in virtualized platforms, we plan to allocate virtual resources (for instance: memory, CPU) by employing simulated annealing.
- Disaster Response Planning:
- In order to improve response durations and resource allocation, the disaster response actions (for example: emergency service implementation) have to be scheduled with the approach of simulated annealing.
- Crowd Management in Public Events:
- Specifically in public phenomena (for instance: sports matches, concerts), handle crowds to improve audience experience and safety. To accomplish this goal, utilize simulated annealing.
- Optimal Service Routing in Telecommunication Networks:
- To enhance service credibility and reduce latency in telecommunication networks, route services in an effective manner through the use of simulated annealing technique.
- Optimal Allocation of Healthcare Resources During Epidemics:
- At the time of epidemics (for example: COVID-19), enhance resource allocation and patient care by allocating healthcare resources with a simulated annealing method.
- Optimal Placement of Charging Stations for Electric Vehicles:
- For reducing charging duration and improving coverage, charging stations have to be deployed by means of simulated annealing, especially for electric vehicles.
- Retail Shelf Space Optimization:
- To decrease inventory expenses and enhance sales, the retail shelf space allocation has to be improved through the utilization of simulated annealing technique.
- Environmental Resource Management:
- By employing the simulated annealing approach, we handle ecological resources (for example: waste management, water distribution), particularly to improve resource effectiveness and viability.
In order to execute simulated annealing in MATLAB, some major procedures are offered by us in an elaborate way. By considering the utilization of simulated annealing in MATLAB, we suggested several intriguing project topics, encompassing outlines in a brief manner. Drop us your project details to get more guidance.
Subscribe Our Youtube Channel
You can Watch all Subjects Matlab & Simulink latest Innovative Project Results
Our services
We want to support Uncompromise Matlab service for all your Requirements Our Reseachers and Technical team keep update the technology for all subjects ,We assure We Meet out Your Needs.
Our Services
- Matlab Research Paper Help
- Matlab assignment help
- Matlab Project Help
- Matlab Homework Help
- Simulink assignment help
- Simulink Project Help
- Simulink Homework Help
- Matlab Research Paper Help
- NS3 Research Paper Help
- Omnet++ Research Paper Help
Our Benefits
- Customised Matlab Assignments
- Global Assignment Knowledge
- Best Assignment Writers
- Certified Matlab Trainers
- Experienced Matlab Developers
- Over 400k+ Satisfied Students
- Ontime support
- Best Price Guarantee
- Plagiarism Free Work
- Correct Citations
Expert Matlab services just 1-click
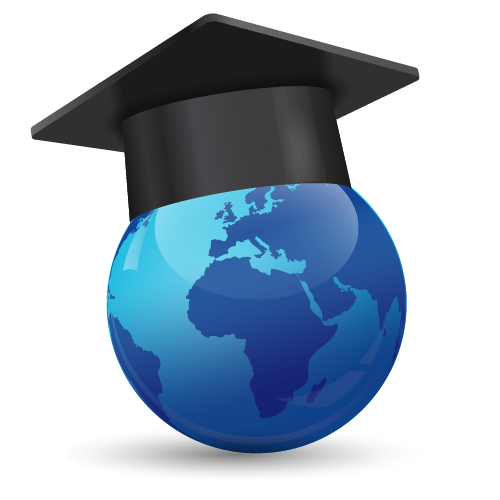
Delivery Materials
Unlimited support we offer you
For better understanding purpose we provide following Materials for all Kind of Research & Assignment & Homework service.
Programs
Designs
Simulations
Results
Graphs
Result snapshot
Video Tutorial
Instructions Profile
Sofware Install Guide
Execution Guidance
Explanations
Implement Plan
Matlab Projects
Matlab projects innovators has laid our steps in all dimension related to math works.Our concern support matlab projects for more than 10 years.Many Research scholars are benefited by our matlab projects service.We are trusted institution who supplies matlab projects for many universities and colleges.
Reasons to choose Matlab Projects .org???
Our Service are widely utilized by Research centers.More than 5000+ Projects & Thesis has been provided by us to Students & Research Scholars. All current mathworks software versions are being updated by us.
Our concern has provided the required solution for all the above mention technical problems required by clients with best Customer Support.
- Novel Idea
- Ontime Delivery
- Best Prices
- Unique Work
Simulation Projects Workflow

Embedded Projects Workflow
