Python Solar Panel Simulation are applied in order to interpret the characteristics of photovoltaic systems, enhance the set up and evaluate their specific functionality, the simulation of solar panels by utilizing Python could be an academic initiative and real-world project. To guide you in designing a solar panel simulation by using Python, we provide step-by-step measures:
Step 1: Install Required Libraries
For visualization, data manipulation and numerical estimations, we can require some efficient Python libraries:
pip install numpy pandas matplotlib
Step 2: Specify Solar Panel Features
Essential features of our solar panel like area, amount of obtained solar radiation and efficacy should be specified by us.
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
# Define constants
efficiency = 0.15 # Efficiency of the solar panel
area = 1.0 # Area of the solar panel in square meters
irradiance = 1000 # Solar irradiance in W/m^2 (standard test conditions)
# Calculate power output
def calculate_power_output(efficiency, area, irradiance):
return efficiency * area * irradiance
power_output = calculate_power_output(efficiency, area, irradiance)
print(f”Power Output: {power_output} W”)
Step 3: Simulate Daily Solar Irradiance
During the day, the divergence of solar irradiance ought to be simulated.
# Simulate solar irradiance over a day
hours = np.arange(0, 24, 1)
daily_irradiance = 1000 * np.sin(np.pi * hours / 24)
plt.plot(hours, daily_irradiance)
plt.xlabel(‘Hour of the Day’)
plt.ylabel(‘Solar Irradiance (W/m^2)’)
plt.title(‘Daily Solar Irradiance’)
plt.grid(True)
plt.show()
Step 4: Estimate Daily Energy Production
Synthesize the power generation output in the course of time to estimate the energy generation throughout a day.
# Calculate power output over the day
daily_power_output = efficiency * area * daily_irradiance
# Calculate energy production (integral of power over time)
energy_production = np.trapz(daily_power_output, hours)
print(f”Daily Energy Production: {energy_production} Wh”)
plt.plot(hours, daily_power_output)
plt.xlabel(‘Hour of the Day’)
plt.ylabel(‘Power Output (W)’)
plt.title(‘Daily Power Output of Solar Panel’)
plt.grid(True)
plt.show()
Step 5: Simulate Monthly and Yearly Energy Production
For several days and months, it is crucial to examine the differences in radiation to estimate the monthly and yearly energy generation by expanding the simulation.
# Simulate monthly irradiance data (assuming a simple sinusoidal variation)
days = np.arange(1, 365 + 1)
monthly_irradiance = 1000 * (0.5 * np.sin(2 * np.pi * (days – 81) / 365) + 0.5)
# Calculate monthly energy production
daily_energy_production = efficiency * area * monthly_irradiance * 24
monthly_energy_production = np.array([np.sum(daily_energy_production[i:i + 30]) for i in range(0, len(days), 30)])
months = np.arange(1, 13)
plt.plot(days, monthly_irradiance)
plt.xlabel(‘Day of the Year’)
plt.ylabel(‘Solar Irradiance (W/m^2)’)
plt.title(‘Yearly Variation of Solar Irradiance’)
plt.grid(True)
plt.show()
plt.bar(months, monthly_energy_production)
plt.xlabel(‘Month’)
plt.ylabel(‘Energy Production (Wh)’)
plt.title(‘Monthly Energy Production’)
plt.grid(True)
plt.show()
# Calculate yearly energy production
yearly_energy_production = np.sum(daily_energy_production)
print(f”Yearly Energy Production: {yearly_energy_production / 1000} kWh”)
Entire Code
In Python, we offer an entire code for simulating a basic solar panel:
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
# Define constants
efficiency = 0.15 # Efficiency of the solar panel
area = 1.0 # Area of the solar panel in square meters
irradiance = 1000 # Solar irradiance in W/m^2 (standard test conditions)
# Calculate power output
def calculate_power_output(efficiency, area, irradiance):
return efficiency * area * irradiance
power_output = calculate_power_output(efficiency, area, irradiance)
print(f”Power Output: {power_output} W”)
# Simulate solar irradiance over a day
hours = np.arange(0, 24, 1)
daily_irradiance = 1000 * np.sin(np.pi * hours / 24)
plt.plot(hours, daily_irradiance)
plt.xlabel(‘Hour of the Day’)
plt.ylabel(‘Solar Irradiance (W/m^2)’)
plt.title(‘Daily Solar Irradiance’)
plt.grid(True)
plt.show()
# Calculate power output over the day
daily_power_output = efficiency * area * daily_irradiance
# Calculate energy production (integral of power over time)
energy_production = np.trapz(daily_power_output, hours)
print(f”Daily Energy Production: {energy_production} Wh”)
plt.plot(hours, daily_power_output)
plt.xlabel(‘Hour of the Day’)
plt.ylabel(‘Power Output (W)’)
plt.title(‘Daily Power Output of Solar Panel’)
plt.grid(True)
plt.show()
# Simulate monthly irradiance data (assuming a simple sinusoidal variation)
days = np.arange(1, 365 + 1)
monthly_irradiance = 1000 * (0.5 * np.sin(2 * np.pi * (days – 81) / 365) + 0.5)
# Calculate monthly energy production
daily_energy_production = efficiency * area * monthly_irradiance * 24
monthly_energy_production = np.array([np.sum(daily_energy_production[i:i + 30]) for i in range(0, len(days), 30)])
months = np.arange(1, 13)
plt.plot(days, monthly_irradiance)
plt.xlabel(‘Day of the Year’)
plt.ylabel(‘Solar Irradiance (W/m^2)’)
plt.title(‘Yearly Variation of Solar Irradiance’)
plt.grid(True)
plt.show()
plt.bar(months, monthly_energy_production)
plt.xlabel(‘Month’)
plt.ylabel(‘Energy Production (Wh)’)
plt.title(‘Monthly Energy Production’)
plt.grid(True)
plt.show()
# Calculate yearly energy production
yearly_energy_production = np.sum(daily_energy_production)
print(f”Yearly Energy Production: {yearly_energy_production / 1000} kWh”)
Python solar panel simulation projects
For simulating a solar panel through the adoption of Python, an extensive group of 100 project concepts are suggested by us that are efficiently capable for performing an intensive research:
- Cloud Cover Implications on Solar Irradiance
- Simulating Solar Irradiance Based on Geographic Location
- Monthly Energy Production Simulation
- Impacts of Orientation (Azimuth) on Solar Panel Efficiency
- Basic Solar Panel Efficiency Simulation
- Temperature Impacts on Solar Panel Performance
- Shading Analysis and Its Effects on Efficiency
- Impact of Panel Tilt Angle on Energy Output
- Daily Power Output Calculation
- Yearly Energy Production Analysis
- Simulation of Solar Panel Degradation over Time
- Simulating Solar Panel Efficiency with Different Inverter Types
- Simulating Solar Panel Performance with Bifacial Panels
- Tracking vs. Fixed Solar Panel Simulation
- Modeling an Optimal Solar Panel Array Layout
- Impacts of Dirt and Dust on Solar Panel Efficiency
- Battery Storage synthesization with Solar Panels
- Comparison of Different Types of Solar Panels (Monocrystalline, Polycrystalline, Thin-film)
- Designing Solar Irradiance Variability over Different Seasons
- Net Metering Simulation
- Simulation of Solar Panel Efficiency with Different Bypass Diode Configurations
- Payback Period Calculation for Solar Installations
- Model and Simulation of Solar-powered Charging Stations
- Implications of Air Pollution on Solar Panel Efficiency
- Performance Analysis of Solar Panels with Reflectors
- Solar Panel Array Performance with various Inter-row Spacings
- Simulation of Solar Panel Performance in Urban vs. Rural Areas
- Return on Investment (ROI) for Solar Panel Systems
- Optimal Placement of Solar Panels on a Roof
- Economic Analysis of Solar Panel Installation
- Utilizing Machine Learning to Predict Solar Panel Output
- Solar Panel Performance Forecasting
- Simulation of Solar Panel Performance with Tracking Algorithms
- Modeling a Solar-powered Water Pumping System
- Simulation of Hybrid Solar-Wind Systems
- Energy Yield Simulation for Various Solar Panel Configurations
- Modeling the Effect of Snow Coverage on Solar Panels
- Simulating Solar Panel Performance for Off-grid Systems
- Real-time Monitoring System for Solar Panels
- Performance Simulation of Floating Solar Panels
- Performance Comparison of Solar Panels in Different Climates
- Optimization of Solar Panel Cleaning Schedules
- Performance Simulation of Solar Panels with Anti-reflective Coatings
- Simulating the Impact of Tree Growth on Solar Panel Shading
- Simulation of Solar Panel Performance in Space Applications
- Modeling Solar-powered Street Lighting Systems
- Simulating Solar Desalination Systems
- Simulation of Solar Power Integration into the Grid
- Designing Solar Thermal Systems
- Modeling Solar-powered Autonomous Vehicles
- Simulating the Impact of Dust Storms on Solar Panel Efficiency
- Solar Panel Performance in Desert Environments
- Modeling the Impacts of Humidity on Solar Panel Performance
- Design and Simulation of Solar-powered Greenhouses
- Modeling the Efficacy of Concentrated Solar Power Systems
- Simulating the Impact of Bird Droppings on Solar Panels
- Energy Simulation for Solar-powered Homes
- Simulation of Solar-powered Irrigation Systems
- Impact of Solar Panel Orientation on Multi-story Buildings
- Simulation of Community Solar Projects
- Modeling Solar Panel Performance with Variable Load Conditions
- Solar Panel Efficiency with Different Types of Glass Covers
- Performance Simulation of Solar Panels with Thermal Management Systems
- Simulation of Solar-powered Emergency Backup Systems
- Designing Solar Panel Systems for Disaster Relief
- Design and Simulation of Solar-powered Boats
- Optimal Design of Solar Panel Mounting Systems
- Designing Solar-powered Air Conditioning Systems
- Simulation of Solar-powered Cooling Systems
- Simulation of Portable Solar Panel Systems
- Simulation of Solar Panels with Integrated Cooling Systems
- Designing Solar-powered Surveillance Systems
- Modeling the Impact of Salt Deposition on Solar Panels
- Simulation of Solar-powered Water Purification Systems
- Performance Comparison of Various Solar Panel Brands
- Simulation of Solar Panel Performance in Coastal Areas
- Impact of Micro-inverters on Solar Panel Efficiency
- Model and Simulation of Solar-powered Agriculture Robots
- Simulation of Solar Panel Performance in High Latitude Regions
- Simulation of Solar-powered Communication Systems
- Modeling Solar Panel Efficiency with Variable Incident Angles
- Implications of Panel Color on Solar Panel Efficiency
- Designing Solar-powered Portable Chargers
- Design and Simulation of Solar-powered Drones
- Performance Simulation of Solar Panels in Foggy Conditions
- Modeling Solar-powered Public Transportation Systems
- Effects of Hail on Solar Panel Durability and Efficiency
- Simulation of Solar-powered Wearable Devices
- Optimization of Solar Panel Maintenance Schedules
- Simulating the Implications of Various Weather Conditions on Solar Panels
- Simulation of Solar Panel Efficiency in Variable Altitudes
- Optimization of Solar Panel Array Configurations for Maximum Efficiency
- Performance Simulation of Solar Panels in Extreme Temperatures
- Simulation of Solar Panel Integration with Smart Grids
- Model and Simulation of Solar-powered Outdoor Furniture
- Simulation of Solar Panel Performance with Dynamic Load Profiles
- Designing the Efficiency of Transparent Solar Panels
- Simulation of Solar-powered Smart Home Systems
- Modeling Solar-powered Health Monitoring Systems
- Simulation of Solar-powered Data Centers.
A simple guide on simulating a solar panel by deploying python is extensively provided by us that guides you effectively throughout the process. In addition to the model program, intriguing project ideas on solar panel simulations are mentioned above.
Explore Python Solar Panel Simulation with our expert help! We work on all the above concepts share your needs, and we will assist you in achieving the best simulation results.
Subscribe Our Youtube Channel
You can Watch all Subjects Matlab & Simulink latest Innovative Project Results
Our services
We want to support Uncompromise Matlab service for all your Requirements Our Reseachers and Technical team keep update the technology for all subjects ,We assure We Meet out Your Needs.
Our Services
- Matlab Research Paper Help
- Matlab assignment help
- Matlab Project Help
- Matlab Homework Help
- Simulink assignment help
- Simulink Project Help
- Simulink Homework Help
- Matlab Research Paper Help
- NS3 Research Paper Help
- Omnet++ Research Paper Help
Our Benefits
- Customised Matlab Assignments
- Global Assignment Knowledge
- Best Assignment Writers
- Certified Matlab Trainers
- Experienced Matlab Developers
- Over 400k+ Satisfied Students
- Ontime support
- Best Price Guarantee
- Plagiarism Free Work
- Correct Citations
Expert Matlab services just 1-click
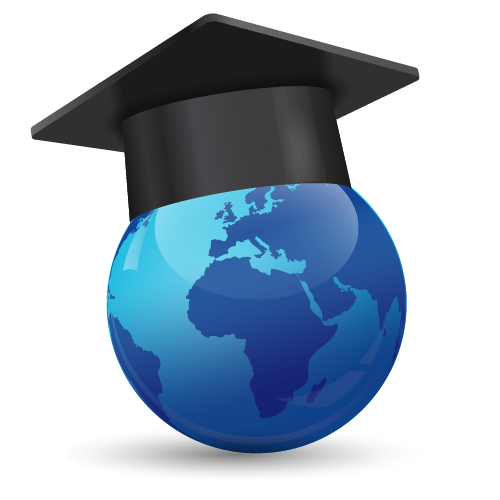
Delivery Materials
Unlimited support we offer you
For better understanding purpose we provide following Materials for all Kind of Research & Assignment & Homework service.
Programs
Designs
Simulations
Results
Graphs
Result snapshot
Video Tutorial
Instructions Profile
Sofware Install Guide
Execution Guidance
Explanations
Implement Plan
Matlab Projects
Matlab projects innovators has laid our steps in all dimension related to math works.Our concern support matlab projects for more than 10 years.Many Research scholars are benefited by our matlab projects service.We are trusted institution who supplies matlab projects for many universities and colleges.
Reasons to choose Matlab Projects .org???
Our Service are widely utilized by Research centers.More than 5000+ Projects & Thesis has been provided by us to Students & Research Scholars. All current mathworks software versions are being updated by us.
Our concern has provided the required solution for all the above mention technical problems required by clients with best Customer Support.
- Novel Idea
- Ontime Delivery
- Best Prices
- Unique Work
Simulation Projects Workflow

Embedded Projects Workflow
