Python Rocket Launch Simulation we share with you several major ideologies like acceleration, velocity, and altitude at the time of launch stage. It can be depicted by a basic rocket launch simulation with Python. To get best Rocket Launch Simulation be in touch with matlabprojects.org we do guarantee best results. Our aim is to support you with clear and understandable Rocket Launch Simulation . Through integrating Newton’s second law of motion, gravity, and air resistance, the dynamics can be designed. Explore rocket simulations with our expert Python help! We offer tailored support to reach your simulation goals. Reach out to matlabprojects.org for professional assistance and the best results. Count on us for timely project delivery.
In order to simulate a rocket launch using Python, we provide a simple instance:
import numpy as np
import matplotlib.pyplot as plt
# Define constants
g = 9.81 # Gravity (m/s^2)
burn_time = 60 # Burn time (seconds)
thrust = 10000 # Thrust force (N)
rocket_mass = 500 # Rocket mass (kg)
fuel_mass = 200 # Fuel mass (kg)
fuel_burn_rate = fuel_mass / burn_time # Fuel consumption rate (kg/s)
drag_coefficient = 0.5 # Coefficient of drag
air_density = 1.225 # Air density at sea level (kg/m^3)
rocket_area = 1.0 # Cross-sectional area of the rocket (m^2)
# Initialize variables
time_step = 0.1 # Time step (seconds)
total_time = 120 # Total simulation time (seconds)
num_steps = int(total_time / time_step)
# Arrays to store simulation data
time_array = np.zeros(num_steps)
altitude_array = np.zeros(num_steps)
velocity_array = np.zeros(num_steps)
acceleration_array = np.zeros(num_steps)
# Initial conditions
altitude = 0
velocity = 0
acceleration = 0
# Run the simulation
for i in range(num_steps):
time = i * time_step
time_array[i] = time
if time < burn_time:
fuel_mass -= fuel_burn_rate * time_step
mass = rocket_mass + fuel_mass
net_force = thrust – mass * g
else:
mass = rocket_mass
net_force = -mass * g # Gravity acts after fuel depletion
# Calculate drag
air_density_at_altitude = air_density * np.exp(-altitude / 10000) # Decreases with altitude
drag_force = 0.5 * drag_coefficient * air_density_at_altitude * rocket_area * velocity**2
net_force -= drag_force
# Update acceleration, velocity, and altitude
acceleration = net_force / mass
velocity += acceleration * time_step
altitude += velocity * time_step
acceleration_array[i] = acceleration
velocity_array[i] = velocity
altitude_array[i] = altitude
# Plot results
plt.figure(figsize=(10, 8))
plt.subplot(3, 1, 1)
plt.plot(time_array, altitude_array)
plt.title(‘Rocket Launch Simulation’)
plt.ylabel(‘Altitude (m)’)
plt.subplot(3, 1, 2)
plt.plot(time_array, velocity_array)
plt.ylabel(‘Velocity (m/s)’)
plt.subplot(3, 1, 3)
plt.plot(time_array, acceleration_array)
plt.xlabel(‘Time (s)’)
plt.ylabel(‘Acceleration (m/s^2)’)
plt.tight_layout()
plt.show()
Description:
- To navigate upwards, the force can be offered by thrust for the rocket.
- As the fuel is consumed, the fuel mass periodically reduces.
- The air resistance (drag) and gravity function in opposition to the launch of a rocket.
- Even though the fuel is drained, the process of simulation executes. This is specifically to demonstrate the impact of gravity on a rocket after the termination of thrust.
Python rocket launch simulation projects
Rocket launch simulation is considered as an intriguing process that involves several procedures and principles. Related to rocket launch, we suggest 50 extensive Python project plans which include various levels of intricacy. From simple physics simulations to highly innovative rocket engineering models, these project plans encompass different topics. For various ranges of skills, every plan can be adapted.
- Basic Rocket Launch Simulation
- Outline: With simple physics concepts such as drag, thrust, and gravity, a basic rocket launch has to be simulated.
- Major Theories: Simple motion equations, gravity, drag, and thrust.
- Rocket Thrust Curve Simulation
- Outline: On the basis of actual engine data, the periodic thrust of a rocket engine must be designed.
- Major Theories: Fuel usage, interpolation of data, and thrust curves.
- 2D Rocket Launch with Wind Resistance
- Outline: At the time of launch, we consider the wind impact on the direction of the rocket and simulate it.
- Major Theories: Fundamental aerodynamics, trajectory adaptation, and wind vectors.
- Rocket Multi-stage Launch
- Outline: Including various stages, a multi-stage rocket should be designed, which fires in a consecutive manner.
- Major Theories: Thrust for each stage, fuel consumption, staging, and mass minimization.
- Rocket with Parachute Recovery System
- Outline: To function at a specific altitude, a parachute recovery approach has to be applied.
- Major Theories: Terminal velocity, descent phase, and parachute motions.
- Rocket Launch to Low Earth Orbit
- Outline: With orbital integration, a rocket launch must be simulated, which attains Low Earth Orbit (LEO).
- Major Theories: Gravity turn activity, delta-v, and orbital mechanics.
- Thrust Vectoring Control Simulation
- Outline: At the time of launch, balance and direct the rocket by applying thrust vector control (TVC).
- Major Theories: Angular momentum, attitude control, and TVC.
- Rocket Launch with Fuel Optimization
- Outline: To reduce time to orbit or increase altitude, we refine the fuel consumption.
- Major Theories: Effectiveness, fuel-to-weight ratio, and optimization methods.
- Real-time Rocket Launch Visualization
- Outline: For a rocket launch, the actual-time 2D or 3D visualization should be developed.
- Major Theories: 2D/3D representation, graphics libraries, and actual-time simulation.
- Simulating Rocket Launch Failure Scenarios
- Outline: Different fault modes have to be designed. It could include parachute fault, structural fault, and engine fault.
- Major Theories: Risk evaluation, system redundancy, and fault conditions.
- Rocket Launch Angle Optimization
- Outline: In terms of physics models, the ideal launch angle has to be estimated for highest altitude.
- Major Theories: Kinematic equations, trajectory enhancement, and angle of launch.
- Rocket Drag and Lift Simulation
- Outline: At the time of flight, the lift and drag forces on the rocket must be designed through the use of actual aerodynamic data.
- Major Theories: Airflow simulation, drag and lift coefficients, and aerodynamics.
- Vertical Rocket Landing Simulation
- Outline: By means of controlled thrust, the vertical landing of a rocket should be simulated.
- Major Theories: Descent regulation, landing legs motions, and reverse thrust.
- Rocket Launch with Real Weather Data
- Outline: To impact the simulation, we utilize actual-time weather data. It could encompass temperature, pressure, and wind speed.
- Major Theories: Wind shear, weather impacts on direction, and API incorporation.
- Simulating Space Shuttle Launch
- Outline: Using firm rocket boosters and major engines, an extensive space shuttle launch must be designed.
- Major Theories: Re-entry, isolation mechanics, and multi-engine simulation.
- Rocket Launch to Escape Velocity
- Outline: A rocket launch has to be simulated, which attains the escape velocity of the Earth.
- Major Theories: Interplanetary travel, energy needs, and escape velocity.
- Rocket Launch with Payload Deployment
- Outline: Focus on designing a rocket launch, which includes particular orbit in satellite placement.
- Major Theories: Satellite placement, orbital integration, and payload dynamics.
- Rocket Engine Design Simulation
- Outline: At the time of launch, we examine the functionality of various rocket engines (liquid, solid, and hybrid) and simulate it.
- Major Theories: Fuel varieties, specific impulse (Isp), and engine categories.
- Rocket Launch and Stage Separation Timing
- Outline: Stage isolation has to be simulated. For isolation, the ideal time must be estimated.
- Major Theories: Timing algorithms and stage isolation dynamics.
- Rocket Launch with Heat Shield Simulation
- Outline: Particularly at the time of ascent and re-entry, the thermal protection framework (heat shield) should be simulated.
- Major Theories: Re-entry dynamics, ablation, and heat transmission.
- Rocket Launch Failure Recovery System
- Outline: A framework has to be applied, in which an automatic recovery series is activated through a rocket fault.
- Major Theories: Recovery techniques, fault identification, and redundancy frameworks.
- Rocket Flight Stability Simulation
- Outline: With fins and gyroscopic stabilization, the flight strength must be simulated.
- Major Theories: Yaw/pitch/roll, gyroscopic regulation, and aerodynamic strength.
- Rocket Launch Data Logger
- Outline: For post-launch exploration, in-depth flight data should be simulated and recorded. It could include acceleration, velocity, and altitude data.
- Major Theories: Flight data exploration, telemetry, and data recording.
- Rocket Launch with Autonomous Guidance
- Outline: In order to adapt the direction in actual time, we apply an automatic guidance framework.
- Major Theories: Guidance algorithms, trajectory rectification, and PID controllers.
- Rocket Launch under Variable Gravity
- Outline: On various celestial objects (Mars and Moon), the rocket launch with diverse gravity has to be simulated.
- Major Theories: Interplanetary tasks, celestial mechanics, and diverse gravity.
- Rocket Launch in Vacuum
- Outline: In a vacuum platform, the launch of a rocket must be simulated. It is important to focus on space states.
- Major Theories: Spaceflight dynamics, vacuum thrust, and no air resistance.
- Rocket Launch in Multibody Environment
- Outline: A rocket launch should be simulated, which is impacted through the several space objects’ gravitational force (Moon and Earth).
- Major Theories: Orbital mechanics, gravitational slingshot, and n-body problem.
- Rocket Launch with Communication Blackouts
- Outline: At the time of re-entry or high-G maneuvers, the interaction outages have to be simulated.
- Major Theories: Telemetry simulation, re-entry outage, and signal loss.
- Rocket Launch with Variable Payload
- Outline: Focus on simulating how rocket functionality and direction are impacted by payload capacity.
- Major Theories: Center of gravity shift, mass impact, and payload dynamics.
- Rocket Launch with G-Force Simulation
- Outline: The g-forces have to be designed, which are confronted by payloads or space travelers at the time of ascent.
- Major Theories: Human aspects in rocket model, acceleration boundaries, and G-force.
- Rocket Launch with Thrust and Drag Optimization
- Outline: For an effective launch, we enhance the thrust-to-drag ratio by applying algorithms.
- Major Theories: Efficiency techniques, thrust enhancement, and drag reduction.
- Rocket Launch with Structural Stress Simulation
- Outline: At various stages of flight, the structural load on the rocket has to be simulated.
- Major Theories: Finite element modeling, stress exploration, and structural mechanics.
- Rocket Launch with Fuel Slosh Simulation
- Outline: Plan to design how the rocket strength and direction are impacted by the motion of liquid fuel (slosh).
- Major Theories: Strength analysis, fluid dynamics, and fuel slosh.
- Rocket Launch with Debris Tracking
- Outline: At the time of fault incidents or stage isolation, the debris must be simulated and monitored.
- Major Theories: Debris monitoring algorithms, collision prevention, and space debris.
- Rocket Engine Efficiency Simulator
- Outline: Various rocket engines have to be simulated. In terms of different fuel varieties, we estimate their effectiveness.
- Major Theories: Fuel-to-thrust ratios, efficiency exploration, and specific impulse.
- Rocket Launch with Electromagnetic Interference (EMI) Simulation
- Outline: During ascent, the EMI impacts on rocket interaction frameworks should be designed.
- Major Theories: Protection methods, signal interruption, and EMI.
- Rocket Launch with Multi-Axis Gimbaling
- Outline: To attain accurate attitude adaptations at the time of launch, the multi-axis gimbal control has to be simulated.
- Major Theories: Feedback frameworks, multi-axis stabilization, and gimbal control.
- Rocket Launch with Space Weather Effects
- Outline: At the time of ascent and in orbit, the space weather impact (radiation and solar flares) on a rocket must be designed.
- Major Theories: Solar wind, radiation shielding, and space weather.
- Rocket Launch from High Altitude
- Outline: A rocket launching from a high-altitude aircraft or balloon should be simulated.
- Major Theories: Ascent phase, atmospheric dynamics, and high-altitude ascent.
- Rocket Launch with Propellant Boil-Off Simulation
- Outline: In what way cryogenic propellants impact task duration and evaporate periodically has to be designed.
- Major Theories: Task planning, propellant boil-off, and cryogenics.
- Rocket Launch under Strong Magnetic Fields
- Outline: Focus on simulating how rocket electronics are impacted by rigid magnetic fields (for instance: close to the poles).
- Major Theories: Navigation problems, electronics protection, and magnetic interference.
- Rocket Launch to Geostationary Orbit
- Outline: A rocket launch has to be simulated, which specifically attains geostationary orbit.
- Major Theories: Altitude targeting, orbital velocity, and geostationary orbit.
- Rocket Launch for Suborbital Tourism
- Outline: For space travel, a suborbital spaceflight must be designed. The launch and descent stages have to be encompassed.
- Major Theories: Descent dynamics, passenger security, and suborbital direction.
- Rocket Launch with Autonomous Landing on a Platform
- Outline: In a moving environment at sea, the automatic landing of a rocket should be simulated.
- Major Theories: Accurate landing, environment dynamics, and automatic landing.
- Simulating a Mars Rocket Launch
- Outline: A launch of a rocket from the Mars region to the Earth surface has to be designed.
- Major Theories: Interplanetary travel, atmosphere, and Mars gravity.
- Rocket Launch with Real-time Telemetry
- Outline: At the time of rocket launch simulation, we intend to stream actual-time telemetry data. It could encompass thrust, velocity, and altitude data.
- Major Theories: Ground control simulation, telemetry, and actual-time data streaming.
- Rocket Launch with Space Debris Avoidance
- Outline: When preventing familiar space debris, examine the rocket’s route and design it.
- Major Theories: Collision identification, trajectory adaptation, and debris prevention.
- Rocket Launch with Reusable Components
- Outline: A rocket launch must be simulated, which includes a few reusable elements (like the initial phase).
- Major Theories: Restoration costs, landing simulations, and reusability.
- Rocket Launch with Solar Sail Deployment
- Outline: A launch of a rocket has to be designed, which facilitates interplanetary travel by implementing a solar sail.
- Major Theories: Interplanetary navigation, propulsion, and solar sail dynamics.
- Rocket Launch with Simulated Communication Latency
- Outline: Among the rocket and task regulation, the impact of interaction delays should be simulated.
- Major Theories: Task control simulation, interaction frameworks, and latency.
For the rocket launch simulation using Python, a simple and explicit instance is offered by us, along with concise descriptions. By emphasizing rocket launch simulation, we recommended numerous compelling Python project plans, including concise outlines and major theories.
Subscribe Our Youtube Channel
You can Watch all Subjects Matlab & Simulink latest Innovative Project Results
Our services
We want to support Uncompromise Matlab service for all your Requirements Our Reseachers and Technical team keep update the technology for all subjects ,We assure We Meet out Your Needs.
Our Services
- Matlab Research Paper Help
- Matlab assignment help
- Matlab Project Help
- Matlab Homework Help
- Simulink assignment help
- Simulink Project Help
- Simulink Homework Help
- Matlab Research Paper Help
- NS3 Research Paper Help
- Omnet++ Research Paper Help
Our Benefits
- Customised Matlab Assignments
- Global Assignment Knowledge
- Best Assignment Writers
- Certified Matlab Trainers
- Experienced Matlab Developers
- Over 400k+ Satisfied Students
- Ontime support
- Best Price Guarantee
- Plagiarism Free Work
- Correct Citations
Expert Matlab services just 1-click
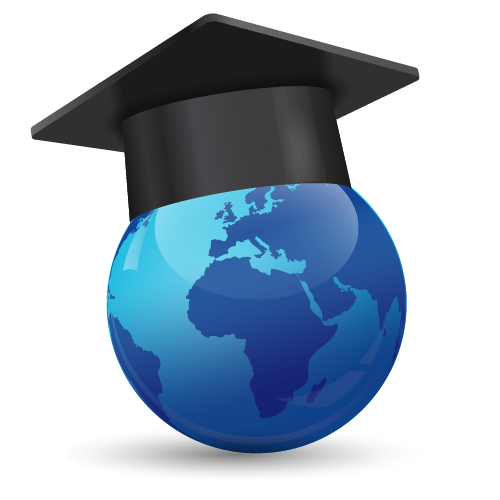
Delivery Materials
Unlimited support we offer you
For better understanding purpose we provide following Materials for all Kind of Research & Assignment & Homework service.
Programs
Designs
Simulations
Results
Graphs
Result snapshot
Video Tutorial
Instructions Profile
Sofware Install Guide
Execution Guidance
Explanations
Implement Plan
Matlab Projects
Matlab projects innovators has laid our steps in all dimension related to math works.Our concern support matlab projects for more than 10 years.Many Research scholars are benefited by our matlab projects service.We are trusted institution who supplies matlab projects for many universities and colleges.
Reasons to choose Matlab Projects .org???
Our Service are widely utilized by Research centers.More than 5000+ Projects & Thesis has been provided by us to Students & Research Scholars. All current mathworks software versions are being updated by us.
Our concern has provided the required solution for all the above mention technical problems required by clients with best Customer Support.
- Novel Idea
- Ontime Delivery
- Best Prices
- Unique Work
Simulation Projects Workflow

Embedded Projects Workflow
