Python Network Traffic Simulation are used for assessing protocols, evaluating the characteristics of networked systems in different scenarios and exploring the network performance, the simulation of network traffic is examined as a useful or beneficial approach. To develop such simulations, Python offer tools with its extensive set of libraries, we have all the needed tools and resources to get your work done on time:
To develop a network traffic simulation with the application of Python, we provide step-by-step measures and instances:
Instance: Simple Network Traffic Simulation
Step 1: Load Required Libraries
Essential libraries have to be imported initially.
import random
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
Step 2: Specify the Network Topology
We have to make use of NetworkX to develop a basic network topology. For developing, exploring and handling complicated networks, NetworkX is highly considered as a dynamic library.
# Create a simple network graph
G = nx.erdos_renyi_graph(10, 0.3) # 10 nodes, probability of edge creation is 0.3
# Draw the network graph
pos = nx.spring_layout(G)
nx.draw(G, pos, with_labels=True, node_color=’lightblue’, node_size=500, font_size=10)
plt.show()
Step 3: Enumerate Traffic Generation and Handling
Among nodes, it is required to simulate traffic generation. Within casually chosen nodes, we consider that the packets are delivered at irregular intervals for convenience.
class NetworkSimulator:
def __init__(self, graph):
self.graph = graph
self.time = 0
self.packet_count = 0
self.traffic_log = []
def generate_packet(self):
src = random.choice(list(self.graph.nodes))
dst = random.choice(list(self.graph.nodes))
if src != dst:
self.packet_count += 1
self.traffic_log.append((self.time, src, dst))
print(f”Time {self.time}: Packet generated from {src} to {dst}”)
def simulate(self, duration, packet_rate):
for t in range(duration):
self.time = t
if random.random() < packet_rate:
self.generate_packet()
# Create a network simulator instance
simulator = NetworkSimulator(G)
Step 4: Execute the Simulation
Within a provided rate of packet generation, we need to execute the simulation for a determined time period.
# Simulate for 100 time units with a packet generation rate of 0.2 per time unit
simulator.simulate(duration=100, packet_rate=0.2)
Step 5: Evaluate the Traffic Data
It is crucial to evaluate the traffic data, once after completing the simulation process. For an instance, in the course of time, the number of packets has to be plotted.
# Extract traffic log data
times, sources, destinations = zip(*simulator.traffic_log)
# Plot the number of packets generated over time
plt.figure()
plt.hist(times, bins=range(101), edgecolor=’black’)
plt.xlabel(‘Time’)
plt.ylabel(‘Number of Packets’)
plt.title(‘Network Traffic Over Time’)
plt.show()
Expanding the Simulation
Note the proceeding developments to enhance the simulation process:
- Routing: To define the path which is occupied by each packet, acquire the benefit of routing algorithms.
- Network Delays: It is required to establish the network delays. The end-to-end latency of packets ought to be evaluated.
- Congestion Control: Congestion control mechanisms are supposed to be simulated and on network functionalities, evaluate the critical effects.
- Failure Scenarios: Network breakdowns such as link failures or nodes have to be simulated and the flexibility of the network should be assessed.
- QoS (Quality of Service): For ranking the specific types of traffic, QoS mechanisms are meant to be executed.
- Traffic Patterns: On the network, examine the critical implications through simulating various traffic patterns like bursty or periodic.
Sample: Including Routing and Delay
To add routing and delay, a basic instance of expanding the simple simulation is offered here:
Specify Routing and Delay Functions
def shortest_path_routing(graph, src, dst):
try:
path = nx.shortest_path(graph, source=src, target=dst)
return path
except nx.NetworkXNoPath:
return []
def simulate_packet_delivery(graph, src, dst):
path = shortest_path_routing(graph, src, dst)
if path:
delay = len(path) – 1
print(f”Packet from {src} to {dst} routed through {path} with delay {delay}”)
return delay
else:
print(f”No path from {src} to {dst}”)
return float(‘inf’)
class NetworkSimulatorWithRouting(NetworkSimulator):
def __init__(self, graph):
super().__init__(graph)
self.total_delay = 0
self.delivered_packets = 0
def generate_packet(self):
src = random.choice(list(self.graph.nodes))
dst = random.choice(list(self.graph.nodes))
if src != dst:
self.packet_count += 1
self.traffic_log.append((self.time, src, dst))
delay = simulate_packet_delivery(self.graph, src, dst)
if delay < float(‘inf’):
self.total_delay += delay
self.delivered_packets += 1
print(f”Time {self.time}: Packet generated from {src} to {dst} with delay {delay}”)
def get_average_delay(self):
if self.delivered_packets > 0:
return self.total_delay / self.delivered_packets
else:
return float(‘inf’)
# Create a network simulator instance with routing
simulator_with_routing = NetworkSimulatorWithRouting(G)
# Simulate for 100 time units with a packet generation rate of 0.2 per time unit
simulator_with_routing.simulate(duration=100, packet_rate=0.2)
# Print average delay
average_delay = simulator_with_routing.get_average_delay()
print(f”Average Packet Delay: {average_delay}”)
Python network traffic simulation projects
By exploring congestion control, traffic generation, routing and more, some of the captivating Python-based projects ideas which cover different perspectives of network simulation are offered here. To offer a thorough interpretation of scope and its probable applications, each project is provided with short specifications and significant features.
- Basic Network Traffic Simulation
- Explanation: In a network, simple network traffic needs to be simulated among nodes. It is approachable to model traffic and scenarios of packet transmission ought to be recorded.
- Main Characteristics: Simple network visualization, packet logging and traffic generation.
- Routing Algorithm Simulation
- Explanation: Diverse routing algorithms in a network such as Bellman-Ford and Dijkstra algorithm are required to be executed and contrasted.
- Main Characteristics: Performance comparison, dynamic routing upgrades and estimation of shortest route.
- Congestion Control Simulation
- Explanation: Congestion control mechanisms like TCP Reno and TCP Tahoe must be simulated. On the basis of network performance, evaluate their crucial implications.
- Main Characteristics: Throughput analysis, congestion window dynamics, retransmission and packet loss.
- Quality of Service (QoS) Simulation
- Explanation: To emphasize various kinds of network traffic and assure quality of service, QoS mechanisms are meant to be utilized.
- Main Characteristics: Priority queuing, bandwidth allocation and traffic classification.
- Network Topology Simulation
- Explanation: Mainly, multiple network topologies like ring, mesh and star have to be simulated and their specific features of functionality is supposed to be assessed.
- Main Characteristics: Performance metrics such as throughput and latency, traffic simulation and generation of topology.
- Wireless Network Simulation
- Explanation: Encompassing the cellular networks and Wi-Fi, wireless networks should be simulated efficiently. The impacts of signal capacity, mobility, and disruptions are required to be evaluated.
- Main Characteristics: Handoff mechanisms, mobility patterns and signal propagation models.
- Peer-to-Peer (P2P) Network Simulation
- Explanation: As a means to examine network efficacy and data distribution, focus on simulating P2P networks and protocols like BitTorrent.
- Main Characteristics: Analysis of network efficacy, data distribution and peer discovery.
- Network Security Simulation
- Explanation: Incorporating defense mechanisms and common assaults such as Man-in-the-Middle and DDoS, network security conditions are meant to be simulated.
- Main Characteristics: Simulation of security protocol, intrusion detection and attack modeling.
- Software-Defined Networking (SDN) Simulation
- Explanation: Considering the centralized network management and regulation, we should explore the advantages by simulating SDN platforms.
- Main Characteristics: Upgrades of dynamic policy, flow table management and execution of SDN controller.
- IoT Network Simulation
- Explanation: For the purpose of investigating network adaptability, data transmission and device responses, IoT (Internet of Things) must be simulated.
- Main Characteristics: Adaptability analysis, communication protocols and IoT device modeling.
- Delay-Tolerant Network (DTN) Simulation
- Explanation: In those networks, it is crucial to examine data transmission and routing.
- Main Characteristics: Routing protocols such as Spray and Wait, Epidemic and Store-and-forward mechanisms.
- Traffic Analysis and Visualization
- Explanation: Network traffic has to be simulated. For evaluating and visualizing traffic patterns, design effective tools.
- Main Characteristics: Visualization dashboards, anomaly detection and traffic monitoring.
- Virtual Private Network (VPN) Simulation
- Explanation: Across public networks, we should investigate authentic communication by simulating VPNs.
- Main Characteristics: Tunnel management, performance analysis and encryption protocols.
- Ad Hoc Network Simulation
- Explanation: Generally, mobile ad hoc networks (MANETs) have to be simulated. Appropriate for decentralized and dynamic platforms, we plan to examine effective routing protocols.
- Main Characteristics: Protocol comparison, dynamic topology variations and mobility frameworks.
- Cloud Network Simulation
- Explanation: It is advisable to examine resource utilization, virtual machine migration and data center networking through simulating the cloud networks.
- Main Characteristics: Network resource management, VM placement and data center topology.
- Network Traffic Optimization
- Explanation: For enhancing the network performance, traffic optimization methods such as load balancing and traffic shaping are required to be executed and simulated by us.
- Main Characteristics: Load balancing algorithms, performance metrics and bandwidth management.
- Vehicular Ad Hoc Network (VANET) Simulation
- Explanation: In order to explore V2I (vehicle-to-infrastructure) and V2V (vehicle-to-vehicle) communication, we need to focus on simulating the VANETs.
- Main Characteristics: Alert message distribution, traffic efficacy and mobility models.
- Network Function Virtualization (NFV) Simulation
- Explanation: As virtualized functions, the evaluation and application of network services must be examined by simulating the platforms of NFV (Network Function Virtualization).
- Main Characteristics: Performance analysis, resource utilization and service chaining.
- Distributed Denial of Service (DDoS) Attack Simulation
- Explanation: Depending on network performance, we have to analyze the effects through simulating DDoS assaults and reduction tactics are required to be assessed.
- Main Characteristics: Reduction methods, detection mechanisms and attack traffic generation.
- Blockchain Network Simulation
- Explanation: Regarding the blockchain protocols, it is approachable to examine the adaptability and functionality by simulating blockchain networks.
- Main Characteristics: Transaction propagation, network latency and consensus algorithms.
Example: Wireless Network Simulation
By utilizing Python, a sample program of basic wireless network simulation is provided below:
Step 1: Load Required Libraries
import random
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
Step 2: Specify the Wireless Network Topology
# Create a simple wireless network graph
G = nx.random_geometric_graph(20, 0.3) # 20 nodes with radius 0.3 for edge creation
# Draw the network graph
pos = nx.get_node_attributes(G, ‘pos’)
nx.draw(G, pos, with_labels=True, node_color=’lightblue’, node_size=500, font_size=10)
plt.show()
Step 3: Enumerate Traffic Generation and Handling
class WirelessNetworkSimulator:
def __init__(self, graph):
self.graph = graph
self.time = 0
self.packet_count = 0
self.traffic_log = []
def generate_packet(self):
src = random.choice(list(self.graph.nodes))
dst = random.choice(list(self.graph.nodes))
if src != dst:
self.packet_count += 1
self.traffic_log.append((self.time, src, dst))
print(f”Time {self.time}: Packet generated from {src} to {dst}”)
def simulate(self, duration, packet_rate):
for t in range(duration):
self.time = t
if random.random() < packet_rate:
self.generate_packet()
# Create a wireless network simulator instance
simulator = WirelessNetworkSimulator(G)
Step 4: Execute the Simulation
# Simulate for 100 time units with a packet generation rate of 0.2 per time unit
simulator.simulate(duration=100, packet_rate=0.2)
Step 5: Evaluate the Traffic Data
# Extract traffic log data
times, sources, destinations = zip(*simulator.traffic_log)
# Plot the number of packets generated over time
plt.figure()
plt.hist(times, bins=range(101), edgecolor=’black’)
plt.xlabel(‘Time’)
plt.ylabel(‘Number of Packets’)
plt.title(‘Wireless Network Traffic Over Time’)
plt.show()
As a means to develop a network traffic simulation with Python, a detailed guide is provided by us with a sample program. Additionally, a set of 20 Python-based network traffic simulation project concepts are extensively discussed with main characteristics and suitable instances.
Drop us a mail we will help you with best simulation results.
Subscribe Our Youtube Channel
You can Watch all Subjects Matlab & Simulink latest Innovative Project Results
Our services
We want to support Uncompromise Matlab service for all your Requirements Our Reseachers and Technical team keep update the technology for all subjects ,We assure We Meet out Your Needs.
Our Services
- Matlab Research Paper Help
- Matlab assignment help
- Matlab Project Help
- Matlab Homework Help
- Simulink assignment help
- Simulink Project Help
- Simulink Homework Help
- Matlab Research Paper Help
- NS3 Research Paper Help
- Omnet++ Research Paper Help
Our Benefits
- Customised Matlab Assignments
- Global Assignment Knowledge
- Best Assignment Writers
- Certified Matlab Trainers
- Experienced Matlab Developers
- Over 400k+ Satisfied Students
- Ontime support
- Best Price Guarantee
- Plagiarism Free Work
- Correct Citations
Expert Matlab services just 1-click
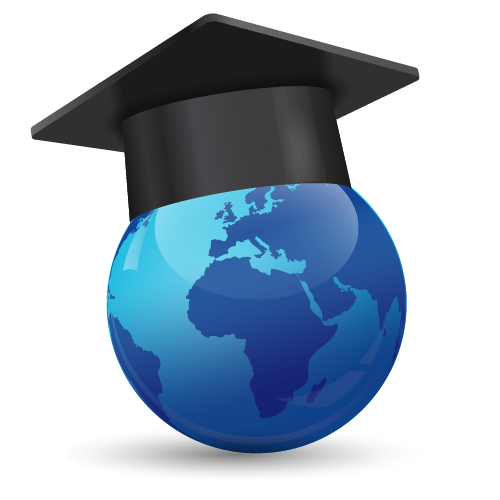
Delivery Materials
Unlimited support we offer you
For better understanding purpose we provide following Materials for all Kind of Research & Assignment & Homework service.
Programs
Designs
Simulations
Results
Graphs
Result snapshot
Video Tutorial
Instructions Profile
Sofware Install Guide
Execution Guidance
Explanations
Implement Plan
Matlab Projects
Matlab projects innovators has laid our steps in all dimension related to math works.Our concern support matlab projects for more than 10 years.Many Research scholars are benefited by our matlab projects service.We are trusted institution who supplies matlab projects for many universities and colleges.
Reasons to choose Matlab Projects .org???
Our Service are widely utilized by Research centers.More than 5000+ Projects & Thesis has been provided by us to Students & Research Scholars. All current mathworks software versions are being updated by us.
Our concern has provided the required solution for all the above mention technical problems required by clients with best Customer Support.
- Novel Idea
- Ontime Delivery
- Best Prices
- Unique Work
Simulation Projects Workflow

Embedded Projects Workflow
