PhD In Python often involves vast libraries that are widely used in existing platforms for developing web applications, modeling techniques and more. Our team are filled with ample resources and team , we have all the research methodologies so we aid you with best guidance across your area with ontime delivery. With the addition of suitable Python libraries which are highly used in PhD studies, some of the important areas of research and competent approaches are offered here:
Important Research Areas and Methods
- Machine Learning and Deep Learning
- Approaches: Convolutional neural networks, supervised learning, unsupervised learning, recurrent neural networks, generative adversarial networks, neural networks and reinforcement learning.
- Significant Libraries: Scikit-learn, Keras, PyTorch and TensorFlow.
- Optimization Algorithms
- Approaches: Integer programming, particle swarm optimization, quadratic programming, linear programming and evolutionary algorithms.
- Significant Libraries: Pyomo, DEAP, CVXPY and PuLP
- Data Mining and Big Data
- Approaches: Large-scale data processing, anomaly detection, clustering and association rule mining.
- Significant Libraries: Apache Spark (PySpark), Scikit-learn, Dask and Pandas.
- Bioinformatics
- Approaches: Gene expression analysis, protein structure prediction, phylogenetics and sequence alignment.
- Significant Libraries: NumPy, SciPy and Biopython.
- Natural Language Processing (NLP)
- Approaches: Named entity recognition, sentiment analysis, machine translation, language models and text classification.
- Significant Libraries: Transformers (by Hugging Face), Gensim, NLTK and SpaCy.
- Computational Geometry
- Approaches: Delaunay triangulation, nearest neighbor search, Voronoi diagrams and convex hull.
- Significant Libraries: CGAL (by means of Python bindings), Shapely and SciPy.
- Computer Vision
- Approaches: Object detection, image segmentation, feature extraction and image classification.
- Significant Libraries: TensorFlow, Keras, PyTorch and OpenCV.
- Graph Algorithms
- Approaches: Minimum spanning tree, graph clustering, maximum flow and shortest path.
- Significant Libraries: Graph-tool, igraph and NetworkX.
- Quantum Computing
- Approaches: Quantum machine learning, quantum algorithms such as Grover’s algorithm, Shor’s algorithm and quantum circuits.
- Significant Libraries: PennyLane, Qiskit and Cirq.
- Simulation and Modeling
- Approaches: Monte Carlo simulations, discrete-event simulation and agent-based modeling.
- Significant Libraries: NumPy, Mesa and SimPy.
Sample Projects and Algorithms
Some of the example projects on Python application are follows:
- Advanced Neural Network Architectures
- For particular applications like natural language interpretation or image creation, we should design and train personalized neural network frameworks.
- Optimization in Logistics
- As a means to enhance logistics networks like supply chain management and vehicle routing, focus on implementing evolutionary algorithms and integer programming.
- Big Data Analytics
- To operate and evaluate terabytes of data, extensive data analysis pipelines have to be executed with the help of Apache Spark.
- Genomic Data Analysis
- By utilizing machine learning, we have to detect mutations, anticipate protein structures and evaluate genomic sequences through modeling efficient algorithms.
- Automated Text Summarization
- It is approachable to design an effective system for outlining the extensive amount of data in an automatic manner by executing advanced NLP methods.
- Efficient Geometric Algorithms
- Especially for geometric problems like GIS (Geographic Information Systems) or collision identification in computer graphics, we need to explore and create effective algorithms.
- Real-time Object Detection
- Acquire the benefit of convolutional neural networks to design real-time object detection systems and on edge devices, implement them.
- Social Network Analysis
- In order to identify groups, detect most significant determinants and evaluate social networks, emphasize on executing the graph algorithms
- Quantum Algorithm Simulation
- Deploy quantum computing libraries and classical computers to simulate and examine quantum algorithms.
- Agent-based Economic Models
- For investigating the economic conditions and economic analysis, agent-based frameworks have to be developed and simulated.
Beginning of Purpose
- Install Required Libraries:
- For our research domain, we have to install the essential libraries by using pip.
pip install numpy scipy pandas scikit-learn tensorflow keras pytorch networkx opencv-python qiskit simpy
- Configure a Programming Platform:
- To configure a responsive programming platform, utilize IDE like PyCharm or Jupyter
- Evaluating Research Papers and Datasets:
- Take advantage of research papers by using environments such as ResearchGate, Google Scholar and arXiv.
- From libraries such as government open data portals, Kaggle and UCI Machine Learning Repository, employ the datasets.
- Work Together and Discuss:
- As a means to version control and teamwork, implement GitHub.
- Share the crucial concepts and acquire reviews by engaging in research organizations and conferences.
Instance of Code Sections
Machine Learning Example (by using Scikit-learn)
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Load dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split data into train and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Train a Random Forest classifier
clf = RandomForestClassifier(n_estimators=100, random_state=42)
clf.fit(X_train, y_train)
# Predict on test set
y_pred = clf.predict(X_test)
# Evaluate accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f’Accuracy: {accuracy * 100:.2f}%’)
Optimization Example (Using PuLP)
import pulp
# Define the problem
problem = pulp.LpProblem(“SimpleLP”, pulp.LpMaximize)
# Define decision variables
x = pulp.LpVariable(‘x’, lowBound=0)
y = pulp.LpVariable(‘y’, lowBound=0)
# Objective function
problem += 3 * x + 2 * y, “Objective”
# Constraints
problem += 2 * x + y <= 20
problem += 4 * x – 5 * y >= -10
problem += x + 2 * y <= 15
# Solve the problem
problem.solve()
# Print the results
print(f”Status: {pulp.LpStatus[problem.status]}”)
print(f”x = {pulp.value(x)}”)
print(f”y = {pulp.value(y)}”)
print(f”Objective = {pulp.value(problem.objective)}”)
Graph Algorithms Example (Using NetworkX)
import networkx as nx
# Create a graph
G = nx.Graph()
# Add nodes and edges
G.add_edges_from([(1, 2), (1, 3), (2, 3), (3, 4)])
# Compute shortest path
shortest_path = nx.shortest_path(G, source=1, target=4)
print(f’Shortest path from 1 to 4: {shortest_path}’)
# Compute clustering coefficient
clustering_coeff = nx.clustering(G)
print(f’Clustering coefficient: {clustering_coeff}’)
Phd in python Research areas
Accompanied with short explanations, core topics and essential tools of specific area, we provide an extensive list of research areas in which Python performs a crucial role that are capable for performing PhD research:
PhD Research projects Using Python
- Machine Learning and Deep Learning
- Explanation: In order to facilitate computers to utilize and create forecasts on data, our team intends to construct and implement effective methods,
- Core Topics: Transfer learning, generative models, reinforcement learning and neural networks.
- Required Tools: PyTorch, Scikit-learn, Keras and TensorFlow.
- Data Science and Big Data Analytics
- Explanation: From extensive datasets, we must make use of statistical and computational methods that effectively retrieve perceptions and intelligence.
- Core Topics: Large-scale data processing, predictive analytics and data mining.
- Required Tools: Apache Spark (PySpark), Dask and Python.
- Natural Language Processing (NLP)
- Explanation: To interpret, recognize and create human language, we need to access the machines by using NLP.
- Core Topics: Sentiment analysis, machine translation, language models and text classification.
- Required Tools: Transformers (by Hugging Face), Gensim, SpaCy and NLTK.
- Computer Vision
- Explanation: For comprehending and explaining visual data from the world, our team intends to create advanced methods.
- Core Topics: Object detection, image segmentation, face recognition and image classification.
- Required Tools: Keras, PyTorch, OpenCV and
- Optimization and Operations Research
- Explanation: Take decisions and address complicated optimization issues through implementing numerical techniques.
- Core Topics: Nonlinear optimization, linear programming, metaheuristics and integer programming.
- Required Tools: SciPy, CVXPY, Pyomo and PuLP.
- Bioinformatics and Computational Biology
- Explanation: With the application of computational methods, we must assess the computational techniques and resolve the complex biological problems in an effective manner.
- Core Topics: Gene expression analysis, protein structure prediction and sequence alignment.
- Required Tools: NumPy, SciPy and Biopython.
- Quantum Computing
- Explanation: Use the primary principles of quantum mechanics to create and evaluate algorithms.
- Core Topics: Quantum circuits, quantum machine learning and quantum algorithms.
- Required Tools: PennyLane, Qiskit and Cirq.
- Robotics and Automation
- Explanation: Carry out tasks in an automatic or semiautomatic manner to model and simulate robots.
- Core Topics: Robot perception, multi-robot systems, control systems and path planning.
- Required Tools: OpenCV, PyBullet and ROS (Robot Operating System).
- Internet of Things (IoT)
- Explanation: To interact and distribute data, the networks of interconnected devices have to be generated and handled efficiently.
- Core Topics: Edge computing, IoT security, smart platforms and sensor networks.
- Required Tools: Raspberry Pi, MQTT and Flask.
- Cybersecurity
- Explanation: From provision of information, impaired or fraud, it is crucial to secure the networks and computer systems.
- Core Topics: Intrusion detection, malware analysis, cryptography and network security.
- Required Tools: OpenSSL, TensorFlow (for anomaly detection), Wireshark and Scapy.
- Financial Engineering and Quantitative Finance
- Explanation: Regarding finance which involves investment tactics and risk management, we need to address the issues with the aid of mathematical frameworks.
- Core Topics: Portfolio optimization, derivatives pricing and algorithmic trading.
- Required Tools: Pandas, QuantLib, NumPy and SciPy.
- Simulation and Modeling
- Explanation: Simulate the complicated operations and systems through the utilization of computational frameworks.
- Core Topics: Monte Carlo simulations, discrete-event simulation and Agent-based modeling.
- Required Tools: NumPy, SimPy and Mesa.
- Human-Computer Interaction (HCI)
- Explanation: Among users and computers, focus on analyzing and modeling the communications.
- Core Topics: Interface model, interaction methods and usability evaluation.
- Required Tools: PyQt, Bokeh and Tkinter.
- Graph Theory and Network Analysis
- Explanation: Mainly, the infrastructure and dynamics of networks must be examined and designed.
- Core Topics: Community detection, network resilience and social network analysis.
- Required Tools: Graph-tool, igraph and NetworkX.
- Environmental and Climate Modeling
- Explanation: To interpret and anticipate ecological and climate variations, consider using computational frameworks.
- Core Topics: Ecological impact evaluation, sustainable resource management and climate simulation.
- Required Tools: SciPy, NumPy and NetCDF4.
- Augmented Reality (AR) and Virtual Reality (VR)
- Explanation: It is approachable to implement AR (Augmented Reality) and VR (Virtual Reality) mechanisms to create captivating experiences.
- Core Topics: User communication, VR simulations and 3D modeling.
- Required Tools: Pygame, PyOpenGL and OpenCV.
- Cognitive Science and AI
- Explanation: Apply AI (Artificial Intelligence) to examine and design thinking capacity of humans.
- Core Topics: Neural representation, learning and memory, and cognitive modeling.
- Required Tools: Keras, PyTorch and TensorFlow.
- Digital Signal Processing (DSP)
- Explanation: Incorporating the image processing and audio processing, digital signals must be evaluated and processed for diverse applications.
- Core Topics: Fourier transforms, wavelet analysis and filtering.
- Required Tools: Librosa, NumPy and SciPy.
- Educational Technology
- Explanation: As a means to improve the procedures of training and learning, create efficient techniques.
- Core Topics: Educational data mining, adaptive learning systems and e-learning environments.
- Required Tools: Pandas, TensorFlow and Jupyter Notebooks.
- Health Informatics
- Explanation: Enhance the healthcare services and medical results, emphasize on the application of information technology on healthcare.
- Core Topics: Health data analytics, electronic health records and telemedicine.
- Required Tools: Scikit-learn, TensorFlow and Pandas.
- Geospatial Analysis
- Explanation: To explore geographic events and correlations, we need to evaluate the geographic data.
- Core Topics: Spatial statistics, GIS and remote sensing.
- Required Tools: Rasterio, Shapely and Geopandas.
- Cryptocurrency and Blockchain
- Explanation: On the basis of blockchain mechanisms and cryptocurrency, our team focuses on creating and evaluating systems.
- Core Topics: Smart contracts, decentralized applications and blockchain protocols.
- Required Tools: PyCrypto, Pandas and Web3.py.
- Energy Systems and Smart Grids
- Explanation: Considering the effective energy management, it is advisable to create and enhance smart grids and energy systems.
- Core Topics: Energy storage, grid flexibility and renewable energy synthesization.
- Required Tools: PyPSA (Python for Power System Analysis) ,SciPy and Pandas.
- Bio-inspired Computing
- Explanation: Algorithms which are influenced through biological systems like neural networks and genetic networks are meant to be designed effectively.
- Core Topics: Swarm intelligence, neural modeling and evolutionary algorithms.
- Required Tools: TensorFlow, Keras and DEAP.
- Social Media Analytics
- Explanation: For the purpose of interpreting user activities and patterns, it is required to assess the social media data.
- Core Topics: Social network analysis, user behavior modeling and sentiment analysis.
- Required Tools: Pandas, Scikit-learn and Tweepy.
Through this article, we provide captivating research concepts on Python with significant approaches, libraries and sample code snippets. Additionally, a set of 25 research areas are suggested above that are useful for performing impactful projects
Subscribe Our Youtube Channel
You can Watch all Subjects Matlab & Simulink latest Innovative Project Results
Our services
We want to support Uncompromise Matlab service for all your Requirements Our Reseachers and Technical team keep update the technology for all subjects ,We assure We Meet out Your Needs.
Our Services
- Matlab Research Paper Help
- Matlab assignment help
- Matlab Project Help
- Matlab Homework Help
- Simulink assignment help
- Simulink Project Help
- Simulink Homework Help
- Matlab Research Paper Help
- NS3 Research Paper Help
- Omnet++ Research Paper Help
Our Benefits
- Customised Matlab Assignments
- Global Assignment Knowledge
- Best Assignment Writers
- Certified Matlab Trainers
- Experienced Matlab Developers
- Over 400k+ Satisfied Students
- Ontime support
- Best Price Guarantee
- Plagiarism Free Work
- Correct Citations
Expert Matlab services just 1-click
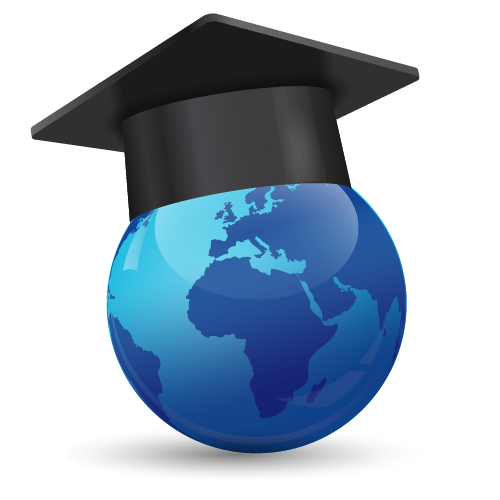
Delivery Materials
Unlimited support we offer you
For better understanding purpose we provide following Materials for all Kind of Research & Assignment & Homework service.
Programs
Designs
Simulations
Results
Graphs
Result snapshot
Video Tutorial
Instructions Profile
Sofware Install Guide
Execution Guidance
Explanations
Implement Plan
Matlab Projects
Matlab projects innovators has laid our steps in all dimension related to math works.Our concern support matlab projects for more than 10 years.Many Research scholars are benefited by our matlab projects service.We are trusted institution who supplies matlab projects for many universities and colleges.
Reasons to choose Matlab Projects .org???
Our Service are widely utilized by Research centers.More than 5000+ Projects & Thesis has been provided by us to Students & Research Scholars. All current mathworks software versions are being updated by us.
Our concern has provided the required solution for all the above mention technical problems required by clients with best Customer Support.
- Novel Idea
- Ontime Delivery
- Best Prices
- Unique Work
Simulation Projects Workflow

Embedded Projects Workflow
