Online Python Code Helper -we at matlabprojects.org will be your best research partner we work on several projects, Python is utilized in combination with ns-3. To enable research in network-related topics, we have suggested an extensive instruction based on how to employ Python along with ns-3 in an effective manner:
Procedural Instruction
- Install ns-3 and ns3-gym:
- To install ns-3, we focus on adhering to the beneficial guidelines.
- For connecting ns-3 with Python, it is advisable to install ns3-gym.
git clone https://gitlab.com/nsnam/ns-3-dev.git
cd ns-3-dev
./waf configure –enable-examples –enable-tests
./waf build
# Install ns3-gym
git clone https://github.com/tkn-tub/ns3-gym.git
cd ns3-gym
pip install .
- Set Up Network Topology in ns-3: In ns-3, our team aims to develop a simple network topology.
// network_topology.cc
#include “ns3/core-module.h”
#include “ns3/network-module.h”
#include “ns3/internet-module.h”
#include “ns3/point-to-point-module.h”
#include “ns3/applications-module.h”
using namespace ns3;
int main(int argc, char *argv[]) {
CommandLine cmd;
cmd.Parse(argc, argv);
NodeContainer nodes;
nodes.Create(2);
PointToPointHelper pointToPoint;
pointToPoint.SetDeviceAttribute(“DataRate”, StringValue(“5Mbps”));
pointToPoint.SetChannelAttribute(“Delay”, StringValue(“2ms”));
NetDeviceContainer devices;
devices = pointToPoint.Install(nodes);
InternetStackHelper stack;
stack.Install(nodes);
Ipv4AddressHelper address;
address.SetBase(“10.1.1.0”, “255.255.255.0”);
Ipv4InterfaceContainer interfaces = address.Assign(devices);
UdpEchoServerHelper echoServer(9);
ApplicationContainer serverApps = echoServer.Install(nodes.Get(1));
serverApps.Start(Seconds(1.0));
serverApps.Stop(Seconds(10.0));
UdpEchoClientHelper echoClient(interfaces.GetAddress(1), 9);
echoClient.SetAttribute(“MaxPackets”, UintegerValue(10));
echoClient.SetAttribute(“Interval”, TimeValue(Seconds(1.0)));
echoClient.SetAttribute(“PacketSize”, UintegerValue(1024));
ApplicationContainer clientApps = echoClient.Install(nodes.Get(0));
clientApps.Start(Seconds(2.0));
clientApps.Stop(Seconds(10.0));
Simulator::Run();
Simulator::Destroy();
return 0;
}
- Create a Python Script for Data Analysis: For machine learning, data analysis, or other research missions, we plan to employ Python.
# data_analysis.py
import pandas as pd
import matplotlib.pyplot as plt
# Example data (replace with actual data collection from ns-3)
data = {
‘Time’: [1, 2, 3, 4, 5],
‘PacketSent’: [100, 200, 300, 400, 500],
‘PacketReceived’: [90, 180, 270, 360, 450],
‘Latency’: [0.1, 0.2, 0.3, 0.4, 0.5]
}
df = pd.DataFrame(data)
# Plot data
plt.figure()
plt.plot(df[‘Time’], df[‘PacketSent’], label=’Packet Sent’)
plt.plot(df[‘Time’], df[‘PacketReceived’], label=’Packet Received’)
plt.xlabel(‘Time (s)’)
plt.ylabel(‘Packets’)
plt.title(‘Network Traffic Over Time’)
plt.legend()
plt.show()
plt.figure()
plt.plot(df[‘Time’], df[‘Latency’], label=’Latency’)
plt.xlabel(‘Time (s)’)
plt.ylabel(‘Latency (s)’)
plt.title(‘Network Latency Over Time’)
plt.legend()
plt.show()
- Integrate ns-3 with Python using ns3-gym: For RL (Reinforcement Learning) or other innovative simulations, construct a Gym platform through the utilization of ns3-gym.
# ns3_gym_interface.py
import gym
import ns3gym
import numpy as np
class Ns3Env(gym.Env):
def __init__(self):
super(Ns3Env, self).__init__()
# Define action and observation space
self.action_space = gym.spaces.Discrete(2)
self.observation_space = gym.spaces.Box(low=0, high=1, shape=(10,), dtype=np.float32)
def step(self, action):
observation = np.random.rand(10)
reward = self.calculate_reward(observation)
done = False
info = {}
return observation, reward, done, info
def reset(self):
return np.random.rand(10)
def calculate_reward(self, observation):
return np.sum(observation) # Example reward function
if __name__ == “__main__”:
env = Ns3Env()
for _ in range(10):
observation = env.reset()
for _ in range(100):
action = env.action_space.sample()
observation, reward, done, info = env.step(action)
if done:
break
- Run the ns-3 Simulation and Python Analysis: We intend to carry out the simulation of ns-3. As a means to process and visualize the outcomes, execute our Python analysis script.
# Build and run the ns-3 simulation
./waf build
./waf –run network_topology
# Run the Python analysis script
python data_analysis.py
online python code services
Python is a multipurpose programming language which can be used in numerous research areas to accomplish a diversity of missions efficiently. We provide a collection of research regions in which Python is utilized:
- Data Structures
- Algorithms
- Computer Networks
- Operating Systems
- Database Management Systems
- Software Engineering
- Digital Logic Design
- Computer Architecture
- Microprocessors
- Embedded Systems
- Signals and Systems
- Control Systems
- Electrical Circuits
- Electronics
- Digital Signal Processing
- Analog Communication
- Digital Communication
- Electromagnetic Theory
- Power Electronics
- Electrical Machines
- Power Systems
- Renewable Energy Systems
- Thermodynamics
- Fluid Mechanics
- Heat Transfer
- Mechanical Vibrations
- Machine Design
- Manufacturing Processes
- Materials Science
- Engineering Mechanics
- Solid Mechanics
- Dynamics
- Kinematics
- Finite Element Analysis
- Robotics
- Mechatronics
- CAD/CAM
- Automobile Engineering
- Aerodynamics
- Aerospace Structures
- Flight Mechanics
- Spacecraft Dynamics
- Chemical Process Engineering
- Chemical Reaction Engineering
- Process Dynamics and Control
- Transport Phenomena
- Biochemical Engineering
- Environmental Engineering
- Water Resources Engineering
- Geotechnical Engineering
- Structural Analysis
- Construction Management
- Surveying and Geomatics
- Transportation Engineering
- Remote Sensing and GIS
- Hydrology
- Urban Planning
- Environmental Impact Assessment
- Bridge Engineering
- Tunnel Engineering
- Railway Engineering
- Airport Engineering
- Coastal Engineering
- Offshore Engineering
- Marine Engineering
- Naval Architecture
- Ship Design
- Ship Construction
- Maritime Safety
- Ocean Engineering
- Petroleum Engineering
- Reservoir Engineering
- Drilling Engineering
- Production Engineering
- Refining and Petrochemicals
- Hydrocarbon Processing
- Subsea Engineering
- Pipeline Engineering
- Nuclear Engineering
- Reactor Physics
- Radiation Protection
- Nuclear Materials
- Nuclear Safety
- Medical Imaging
- Biomechanics
- Biomaterials
- Tissue Engineering
- Bioinformatics
- Genomics
- Proteomics
- Systems Biology
- Biostatistics
- Biomedical Instrumentation
- Clinical Engineering
- Rehabilitation Engineering
- Pharmaceutical Engineering
- Polymer Science
- Nanotechnology
- Material Characterization
- Surface Engineering
- Corrosion Engineering
- Composite Materials
- Smart Materials
- Metallurgy
- Mineral Processing
- Extractive Metallurgy
- Steel Making
- Non-Ferrous Metals
- Welding Technology
- Ceramics Engineering
- Glass Technology
- Semiconductor Devices
- Photonics
- Optoelectronics
- Laser Technology
- Quantum Computing
- Cryptography
- Network Security
- Cybersecurity
- Artificial Intelligence
- Machine Learning
- Deep Learning
- Natural Language Processing
- Computer Vision
- Data Mining
- Big Data Analytics
- Cloud Computing
- Internet of Things
- Blockchain Technology
- Virtual Reality
- Augmented Reality
- Human-Computer Interaction
- Robotics Process Automation
- Digital Twins
- Smart Grids
- Industrial Automation
- Sustainable Engineering
- Green Technology
- Renewable Energy Systems
- Energy Storage Systems
- Electric Vehicles
- Smart Cities
- Autonomous Vehicles
Through this article, we have recommended a thorough direction on the basis of how you could employ Python together with ns-3 to promote research in network-based topics. Also, a list of research areas in which Python is utilized are offered by us in an explicit manner.
Distinctive step-by-step instructions are provided for your tasks. Seek assistance from Python specialists to address your coding needs at matlabprojects.org. Submit your project details to us for optimal coding outcomes.
Subscribe Our Youtube Channel
You can Watch all Subjects Matlab & Simulink latest Innovative Project Results
Our services
We want to support Uncompromise Matlab service for all your Requirements Our Reseachers and Technical team keep update the technology for all subjects ,We assure We Meet out Your Needs.
Our Services
- Matlab Research Paper Help
- Matlab assignment help
- Matlab Project Help
- Matlab Homework Help
- Simulink assignment help
- Simulink Project Help
- Simulink Homework Help
- Matlab Research Paper Help
- NS3 Research Paper Help
- Omnet++ Research Paper Help
Our Benefits
- Customised Matlab Assignments
- Global Assignment Knowledge
- Best Assignment Writers
- Certified Matlab Trainers
- Experienced Matlab Developers
- Over 400k+ Satisfied Students
- Ontime support
- Best Price Guarantee
- Plagiarism Free Work
- Correct Citations
Expert Matlab services just 1-click
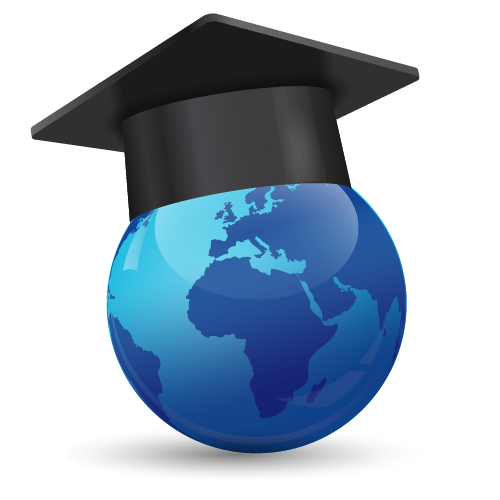
Delivery Materials
Unlimited support we offer you
For better understanding purpose we provide following Materials for all Kind of Research & Assignment & Homework service.
Programs
Designs
Simulations
Results
Graphs
Result snapshot
Video Tutorial
Instructions Profile
Sofware Install Guide
Execution Guidance
Explanations
Implement Plan
Matlab Projects
Matlab projects innovators has laid our steps in all dimension related to math works.Our concern support matlab projects for more than 10 years.Many Research scholars are benefited by our matlab projects service.We are trusted institution who supplies matlab projects for many universities and colleges.
Reasons to choose Matlab Projects .org???
Our Service are widely utilized by Research centers.More than 5000+ Projects & Thesis has been provided by us to Students & Research Scholars. All current mathworks software versions are being updated by us.
Our concern has provided the required solution for all the above mention technical problems required by clients with best Customer Support.
- Novel Idea
- Ontime Delivery
- Best Prices
- Unique Work
Simulation Projects Workflow

Embedded Projects Workflow
