Ant Colony Optimization MATLAB project and topic ideas are listed in this page, we provide you best simulation results for your projects. You have to do is share with us your project details for more guidance. ACO stands for Ant Colony Optimization which is prevalently used metaheuristic technique. Searching behavior of ants was the major inspiration of this method. For addressing integrative optimization issues like TSP (Traveling Salesman Problem), this technique is highly applicable. To execute ACO for the TSP in MATLAB, we offer a considerable instance:
Key Concept
- Initialization: On entire paths, begin with pheromone levels.
- Ant Movement: Depending on empirical details and pheromone levels, each ant moves randomly to the next city to develop efficient findings.
- Pheromone Upgrades: According to the standard of the solutions which are detected by ants, the pheromone levels should be upgraded.
- Iteration: Until integration or definite number of attempts, the processes need to be reiterated.
MATLAB Execution
Considering the TSP (Traveling Salesman Problem), a simple execution of ACO is offered below:
% Ant Colony Optimization for Traveling Salesman Problem
clc; clear; close all;
% Parameters
nCities = 10; % Number of cities
nAnts = 20; % Number of ants
nIterations = 100; % Number of iterations
alpha = 1; % Pheromone importance
beta = 5; % Heuristic importance
rho = 0.5; % Evaporation rate
Q = 100; % Pheromone increase constant
% Generate random cities
cities = rand(nCities, 2);
distanceMatrix = squareform(pdist(cities));
% Initialize pheromone levels
pheromoneMatrix = ones(nCities);
% Ant Colony Optimization loop
bestTour = [];
bestTourLength = Inf;
for iter = 1:nIterations
tours = zeros(nAnts, nCities);
tourLengths = zeros(nAnts, 1);
for ant = 1:nAnts
% Randomly choose the starting city
startCity = randi(nCities);
tours(ant, 1) = startCity;
visited = false(1, nCities);
visited(startCity) = true;
for step = 2:nCities
currentCity = tours(ant, step-1);
% Calculate probabilities for next city
probabilities = zeros(1, nCities);
for city = 1:nCities
if ~visited(city)
probabilities(city) = (pheromoneMatrix(currentCity, city)^alpha) * …
((1 / distanceMatrix(currentCity, city))^beta);
end
end
probabilities = probabilities / sum(probabilities);
% Roulette wheel selection
nextCity = find(rand <= cumsum(probabilities), 1);
tours(ant, step) = nextCity;
visited(nextCity) = true;
end
% Calculate tour length
tourLength = 0;
for step = 1:(nCities-1)
tourLength = tourLength + distanceMatrix(tours(ant, step), tours(ant, step+1));
end
tourLength = tourLength + distanceMatrix(tours(ant, end), tours(ant, 1)); % Return to start
tourLengths(ant) = tourLength;
% Update best tour
if tourLength < bestTourLength
bestTourLength = tourLength;
bestTour = tours(ant, :);
end
end
% Update pheromone levels
pheromoneMatrix = (1 – rho) * pheromoneMatrix; % Evaporation
for ant = 1:nAnts
for step = 1:(nCities-1)
pheromoneMatrix(tours(ant, step), tours(ant, step+1)) = …
pheromoneMatrix(tours(ant, step), tours(ant, step+1)) + Q / tourLengths(ant);
end
pheromoneMatrix(tours(ant, end), tours(ant, 1)) = …
pheromoneMatrix(tours(ant, end), tours(ant, 1)) + Q / tourLengths(ant);
end
% Display progress
disp([‘Iteration ‘, num2str(iter), ‘: Best Tour Length = ‘, num2str(bestTourLength)]);
end
% Plot best tour
figure;
plot(cities(bestTour, 1), cities(bestTour, 2), ‘o-‘, ‘LineWidth’, 2);
hold on;
plot([cities(bestTour(end), 1), cities(bestTour(1), 1)], …
[cities(bestTour(end), 2), cities(bestTour(1), 2)], ‘o-‘, ‘LineWidth’, 2);
title([‘Best Tour Length: ‘, num2str(bestTourLength)]);
xlabel(‘X’);
ylabel(‘Y’);
grid on;
% Functions
function dist = pdist(cities)
dist = sqrt((cities(:,1) – cities(:,1)’).^2 + (cities(:,2) – cities(:,2)’).^2);
end
Description
- Initialization: Cities are created in a probable manner, distance matrix is estimated and up to 1, the Pheromone levels are computed.
- Ant Movement: In accordance with pheromone phases and inverse distances, each ant selects the next city randomly by organizing a tour.
- Pheromone Upgrades: On the basis of duration of tour, the Pheromone phases are upgraded. Extensive pheromones are contributed by means of optimal tours.
- Iteration: For a particular number of attempts, the process must be reiterated.
Significant Parameters
- nCities: Generally in the TSP, it represents the number of cities.
- nAnts: It denotes the number of ants which are deployed in the technique.
- nIterations: In executing the techniques, the number of repetitions are determined.
- alpha: The relevance of pheromone levels is exhibited here.
- beta: It reflects the significance of empirical data (inverse of distance).
- rho: Considering the pheromone, the evaporation rate is determined.
- Q: This indicates pheromone deposit constant.
Important 75 ant colony optimization algorithms list
ACO (Ant Colony Optimization) efficiently decreases the cost of maintenance and enhances the service quality. Including field-specific applications, hybrid techniques, various optimization issues and developments in architecture of algorithms, numerous remarkable techniques of colony optimization are proposed by us:
Common Diversities and Developments
- Dynamic Ant Colony Optimization
- ACO with Memetic techniques
- ACO with Evolutionary tactics
- ACO with Reinforcement Learning
- ACO with Differential Evolution
- Hybrid Ant Colony Optimization
- Adaptive Ant Colony Optimization
- Parallel Ant Colony Optimization
- ACO with Genetic Algorithms
- ACO with Simulated Annealing
- ACO with Particle Swarm Optimization (PSO)
- ACO with Tabu Search
- Basic Ant Colony Optimization (ACO)
- Elitist Ant System
- Continuous Ant Colony Optimization
- ACO with Local Search
- Max-Min Ant System (MMAS)
- Rank-Based Ant System
- Multi-Colony ACO
- Ant Colony System (ACS)
Critical Applications in Combinatorial Optimization
- Periodic Vehicle Routing Problem
- Dial-a-Ride Problem
- Stochastic Vehicle Routing Problem
- Cutting Stock Problem
- Travelling Thief Problem (TTP)
- Capacitated Vehicle Routing Problem
- Traveling Salesman Problem (TSP)
- Bin Packing Problem
- Set Covering Problem
- Assembly Line Balancing Problem
- Inventory Management Problem
- Quadratic Assignment Problem (QAP)
- Multi-Depot Vehicle Routing Problem
- Minimum Spanning Tree Problem
- Shortest Path Problem
- Facility Location Problem
- Vehicle Routing Problem (VRP)
- Job Shop Scheduling Problem (JSSP)
- Maximum Clique Problem
- Graph Coloring Problem
- Network Design Problem
- Knapsack Problem
Critical Applications in Network Optimization
- Network Congestion Control
- Network Intrusion Detection
- Load Balancing in Networks
- Sensor Network Optimization
- QoS Routing in Networks
- Ad-Hoc Wireless Network Routing
- Network Routing
- Multicast Routing
- Internet Packet Routing
Critical Applications in Control and Robotics
- Unmanned Aerial Vehicle (UAV) Path Planning
- Autonomous Vehicle Routing
- Swarm Robotics
- Robot Coordination
- Robot Localization
- Robot Path Planning
Critical Applications in Bioinformatics
- Gene Regulatory Network Inference
- Genome Assembly
- Protein Folding
- Protein-Ligand Docking
- DNA Sequencing
Critical Applications in Engineering Design
- Antenna Design
- Power System Optimization
- Control System Design
- Structural Optimization
- Electrical Circuit Design
Critical Applications in Image and Signal Processing
- Signal Filtering
- Speech Recognition
- Image Edge Detection
- Image Matching
- Image Segmentation
Critical Applications in Financial Optimization
- Financial Forecasting
- Algorithmic Trading
- Portfolio Optimization
For addressing the optimization issues, ACO plays a crucial role with its efficient capabilities. A simple instance of executing ACO for TSP in MATLAB and also some of the crucial algorithms which are applicable in diverse areas are provided in this article. Drop us your parameter details for best guidance. We help you in your project execution with best thesis writing services.
Subscribe Our Youtube Channel
You can Watch all Subjects Matlab & Simulink latest Innovative Project Results
Our services
We want to support Uncompromise Matlab service for all your Requirements Our Reseachers and Technical team keep update the technology for all subjects ,We assure We Meet out Your Needs.
Our Services
- Matlab Research Paper Help
- Matlab assignment help
- Matlab Project Help
- Matlab Homework Help
- Simulink assignment help
- Simulink Project Help
- Simulink Homework Help
- Matlab Research Paper Help
- NS3 Research Paper Help
- Omnet++ Research Paper Help
Our Benefits
- Customised Matlab Assignments
- Global Assignment Knowledge
- Best Assignment Writers
- Certified Matlab Trainers
- Experienced Matlab Developers
- Over 400k+ Satisfied Students
- Ontime support
- Best Price Guarantee
- Plagiarism Free Work
- Correct Citations
Expert Matlab services just 1-click
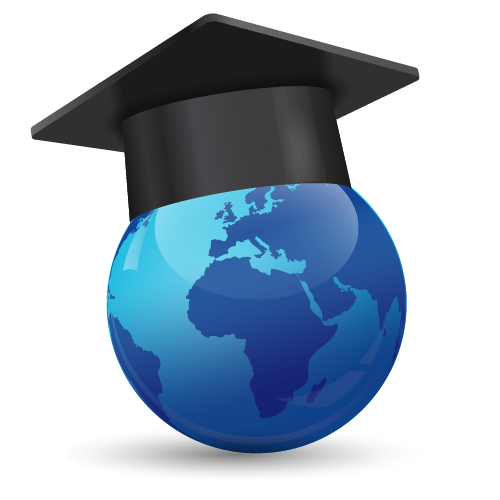
Delivery Materials
Unlimited support we offer you
For better understanding purpose we provide following Materials for all Kind of Research & Assignment & Homework service.
Programs
Designs
Simulations
Results
Graphs
Result snapshot
Video Tutorial
Instructions Profile
Sofware Install Guide
Execution Guidance
Explanations
Implement Plan
Matlab Projects
Matlab projects innovators has laid our steps in all dimension related to math works.Our concern support matlab projects for more than 10 years.Many Research scholars are benefited by our matlab projects service.We are trusted institution who supplies matlab projects for many universities and colleges.
Reasons to choose Matlab Projects .org???
Our Service are widely utilized by Research centers.More than 5000+ Projects & Thesis has been provided by us to Students & Research Scholars. All current mathworks software versions are being updated by us.
Our concern has provided the required solution for all the above mention technical problems required by clients with best Customer Support.
- Novel Idea
- Ontime Delivery
- Best Prices
- Unique Work
Simulation Projects Workflow

Embedded Projects Workflow
