Python Pendulum Simulation is a typical instance of resolving differential equations. It is the process of simulating a pendulum in Python. Encompassing numerical integration techniques, there are numerous methods which you could employ. We provide a basic instance of utilizing the Runge-Kutta method (RK4) to simulate a basic pendulum effectively:
Problem Configuration
Generally, for a basic pendulum, the equation of motion can be indicated as:
d2θdt2+gLsin(θ)=0\frac{d^2 \theta}{dt^2} + \frac{g}{L} \sin(\theta) = 0dt2d2θ+Lgsin(θ)=0
Where:
- The angle of the pendulum is denoted as θ\thetaθ.
- Typically, ggg indicates the acceleration because of gravity.
- The length of the pendulum is specified as LLL.
For numerical incorporation, we focus on transforming this second-order differential equation into a system of first-order differential equations.
Procedures to Simulate a Pendulum
- Define the System of Equations: Mainly, the second-order differential equation must be transformed into a system of first-order equations.
- Numerical Integration: As a means to resolve the system of equations, it is beneficial to employ an integration technique.
- Visualization: To visualize the movement of the pendulum, we plan to plot the outcomes.
Python Implementation
The following is a Python script which simulates the movement of a basic pendulum with the support of the Runge-Kutta technique:
import numpy as np
import matplotlib.pyplot as plt
# Constants
g = 9.81 # acceleration due to gravity (m/s^2)
L = 1.0 # length of the pendulum (m)
# Define the system of differential equations
def pendulum_derivs(y, t):
theta, omega = y
dydt = [omega, – (g / L) * np.sin(theta)]
return dydt
# Time settings
t_start = 0.0
t_end = 10.0
dt = 0.01
t = np.arange(t_start, t_end, dt)
# Initial conditions
theta0 = np.pi / 4 # initial angle (radians)
omega0 = 0.0 # initial angular velocity
y0 = [theta0, omega0]
# Solve the differential equations
from scipy.integrate import odeint
solution = odeint(pendulum_derivs, y0, t)
theta, omega = solution[:, 0], solution[:, 1]
# Plot the results
plt.figure(figsize=(10, 5))
plt.subplot(1, 2, 1)
plt.plot(t, theta)
plt.xlabel(‘Time (s)’)
plt.ylabel(‘Theta (rad)’)
plt.title(‘Pendulum Angle vs Time’)
plt.subplot(1, 2, 2)
plt.plot(theta, omega)
plt.xlabel(‘Theta (rad)’)
plt.ylabel(‘Omega (rad/s)’)
plt.title(‘Phase Space Plot’)
plt.tight_layout()
plt.show()
Description
- pendulum_derivs: The system of first-order differential equations has to be described.
- odeint: In order to incorporate the system of equations, it is advisable to make use of the odeint function from SciPy.
- Visualization: Periodically, the pendulum angle ought to be plotted. For demonstrating the connection among angular velocity and angle, focus on offering phase space plot.
python pendulum simulation projects
If you are choosing a project topic on a pendulum simulation using Python, you must prefer impactful and significant project topics. To guide you in this process, by offering possibilities to investigate various factors of pendulum dynamics and simulation approaches, we suggest few projects that differ in range and complication:
- Basic Pendulum Simulation: By means of employing simple physics equations, we focus on simulating a basic pendulum.
- Damped Pendulum: As a means to investigate loss of energy, our team aims to append damping to the pendulum simulation.
- Driven Pendulum: A pendulum ought to be simulated which is influenced by an external periodic force.
- Double Pendulum: The disruptive features of a double pendulum should be designed.
- Pendulum with Air Resistance: In the simulation, we intend to encompass impacts of air resistance.
- Pendulum with Variable Length: Generally, a pendulum should be simulated in which a length varies periodically.
- Pendulum with Variable Gravity: In differing gravitational fields, our team plans to design a pendulum.
- Pendulum with Nonlinear Damping: On the movement of the pendulum, it is significant to examine the impacts of nonlinear damping.
- Pendulum in a Rotating Frame: In a rotating reference frame, we focus on simulating a pendulum.
- Inverted Pendulum: An inverted pendulum must be designed. It is advisable to explore its flexibility.
- Pendulum with External Torque: On the pendulum, our team aims to simulate the impacts of an external torque.
- Interactive Pendulum Simulation: An interactive simulation ought to be developed in which users are able to adapt to metrics such as gravity and length.
- Pendulum on a Moving Cart: A pendulum must be simulated which is connected to a cart that moves in a horizontal manner.
- Pendulum with Variable Mass: On the movement of the pendulum, we plan to investigate the impacts of varying mass.
- Pendulum with Magnetic Forces: In the pendulum simulation, it is appreciable to encompass magnetic forces.
- Pendulum with Spring Attachment: A spring has to be connected to the pendulum. Our team intends to simulate its characteristics.
- Pendulum with Friction: To the pivot point of the pendulum, we aim to append friction.
- Pendulum with Energy Dissipation: In the pendulum model, it is significant to design dissipation of energy.
- Multiple Coupled Pendulums: A system of coupled pendulums is required to be simulated.
- Pendulum with Feedback Control: As a means to balance a pendulum, our team applies feedback control.
- Pendulum in a Fluid: A pendulum traveling across a viscous fluid should be simulated.
- Pendulum with Thermal Effects: On the pendulum, we explore the impacts of temperature variations.
- Pendulum with Adaptive Length: Focus on simulating a pendulum in which length can be adjusted on the basis of specific measures.
- Pendulum Simulation with GUI: To communicate with the pendulum simulation, we focus on creating a graphical user interface.
- Pendulum with Random Perturbations: In order to examine impacts of noise, it is approachable to include random perturbations.
- Pendulum in a Non-Uniform Gravitational Field: In an inconsistent gravitational field, our team designs a pendulum.
- Pendulum with Rotating Pivot: A pendulum with pivot point which revolves must be simulated.
- Pendulum with Stochastic Forces: To the pendulum model, we aim to initiate stochastic forces.
- 3D Pendulum Simulation: Typically, the pendulum simulation must be prolonged to three dimensions.
- Pendulum with Multiple Degrees of Freedom: Including highly intricate degrees of freedom, a pendulum has to be designed.
- Pendulum with Adjustable Amplitude: On the movement of the pendulum, it is appreciable to examine the impacts of differing amplitude.
- Pendulum with Time-Varying Parameters: By encompassing parameters which vary periodically, a pendulum ought to be simulated.
- Pendulum with Complex Initial Conditions: Generally, different complicated initial scenarios and their impacts have to be investigated.
- Pendulum Simulation with Numerical Stability Analysis: We intend to examine the numerical flexibility of various integration techniques.
- Pendulum with Multiple Couplings: In the model, our team examines the impacts of numerous coupling technologies.
- Pendulum with Nonlinear Restoring Forces: In the simulation, it is advisable to involve nonlinear restoring forces.
- Pendulum with Custom Forces: In order to investigate specific characteristics, our team aims to apply conventional force operations.
- Pendulum with Control Algorithms: To alter or balance the movement of the pendulum, we intend to implement various control methods.
- Pendulum with Real-Time Data Visualization: For the movement of the pendulum, it is appreciable to develop actual time data visualizations.
- Pendulum with Feedback Linearization: As a means to regulate the pendulum, our team utilizes feedback linearization approaches.
- Pendulum with Adaptive Control Strategies: For pendulum stability, we focus on examining adaptive control policies.
- Pendulum with Lyapunov Stability Analysis: Through the utilization of Lyapunov techniques, examine the flexibility of the pendulum.
- Pendulum with Particle Swarm Optimization: To identify the optimum metrics for the pendulum, it is beneficial to employ particle swarm optimization.
- Pendulum with Genetic Algorithms: For reinforcing pendulum metrics, we implement genetic methods.
- Pendulum with Neural Network Control: Typically, neural network-related control tactics should be applied.
- Pendulum with Reinforcement Learning: For regulation, our team intends to implement reinforcement learning approaches.
- Pendulum with Simulated Sensor Data: For actual time regulation, we plan to combine simulated sensor data.
- Pendulum with Sensor Fusion: To enhance control authenticity, it is significant to apply sensor fusion methods.
- Pendulum with Machine Learning Models: As a means to forecast and manage pendulum movement, our team intends to utilize machine learning systems.
- Pendulum with Kalman Filtering: For state assessment and management, focus on implementing Kalman filtering.
- Pendulum with Particle Filter: Generally, particle filtering must be applied for state evaluation.
- Pendulum with Monte Carlo Methods: Under ambiguity, examine pendulum characteristics through the utilization of Monte Carlo techniques.
- Pendulum with Hybrid Control Systems: Hybrid control models which integrate various methods should be examined intensively.
- Pendulum with Robotic Arm Integration: Typically, a pendulum incorporated with a robotic arm has to be simulated.
- Pendulum with Multi-Agent Systems: Encompassing the diverse pendulums, we need to explore the multi-agent systems.
- Pendulum with Optimal Control Theory: In order to identify the efficient control policies, it is appreciable to implement optimum control concept.
- Pendulum with Adaptive Learning Rate: In control methods, we apply adaptive learning rates.
- Pendulum with Real-World Data Calibration: With the aid of actual world pendulum data, focus on adjusting the simulation.
- Pendulum with Simulated Environments: To examine characteristics of pendulum, we plan to develop various simulated platforms.
- Pendulum with Haptic Feedback: For communicative simulations, it is advisable to apply haptic feedback.
- Pendulum with Virtual Reality Integration: In virtual reality platforms, our team aims to investigate pendulum simulations.
- Pendulum with Augmented Reality Visualization: As a means to visualize the movement of the pendulum, we employ augmented reality.
- Pendulum with Multi-Scale Modeling: At various levels of observation, it is significant to investigate the pendulum.
- Pendulum with Hybrid Simulation Techniques: For improved precision, our team incorporates various simulation approaches.
- Pendulum with Event-Driven Simulation: Specifically, for discrete events, focus on applying event-driven simulation.
- Pendulum with Computational Fluid Dynamics (CFD): The CFD must be combined for extensive fluid impacts.
- Pendulum with Structural Dynamics Analysis: Mainly, structural dynamics impacting the pendulum ought to be examined.
- Pendulum with High-Performance Computing: For extensive simulations, we intend to employ high-effective computing resources.
- Pendulum with Distributed Simulation: For extensive models, it is appreciable to apply distributed simulation methods.
- Pendulum with Parallel Computing: To attain rapid simulations, parallel computing ought to be examined effectively.
- Pendulum with Cloud-Based Simulation: For pendulum simulations, our team plans to utilize cloud computing resources.
- Pendulum with Real-Time Monitoring: Generally, for pendulum characteristics, carry out actual time tracking and exploration.
- Pendulum with Online Learning: In order to adjust control policies, it is advisable to implement online learning approaches.
- Pendulum with IoT Integration: For remote control and tracking, focus on combining IoT devices.
- Pendulum with Automated Testing: Generally, automated testing models have to be constructed for pendulum simulations.
- Pendulum with Experimental Validation: By means of empirical data, we verify simulation outcomes.
- Pendulum with Error Analysis: On simulation precision, our team aims to investigate the influence of numerical faults.
- Pendulum with Sensitivity Analysis: As a means to interpret parameter influences, it is appreciable to carry out the process of sensitivity analysis.
- Pendulum with Robust Control Design: For pendulum stability, we plan to model efficient control models.
- Pendulum with Fault Tolerance: Typically, fault-tolerant models must be applied for pendulum management.
- Pendulum with Multiple Pendulum Systems: In a model, simulate and manage numerous pendulums in an effective manner.
- Pendulum with Nonlinear Control Techniques: For pendulum stability, our team intends to examine nonlinear control techniques.
- Pendulum with Dynamic Simulation: Periodically, we explore dynamic variations in pendulum models.
- Pendulum with Non-Equilibrium Analysis: The pendulum characteristics with equilibrium conditions has to be examined.
- Pendulum with Predictive Modeling: To predict movement of the pendulum, it is beneficial to utilize predictive modeling methods.
- Pendulum with System Identification: In order to design pendulum dynamics, we aim to apply system identification approaches.
- Pendulum with Fault Detection: For identifying mistakes in pendulum models, our team creates effective techniques.
- Pendulum with Optimization-Based Control: To manage pendulum characteristics, it is advisable to implement optimization approaches.
- Pendulum with Multi-Objective Optimization: For pendulum management, we investigate multi-objective optimization.
- Pendulum with Real-Time Feedback Systems: Mainly, for regulation, apply actual time feedback models.
- Pendulum with Adaptive Systems: For varying pendulum metrics, our team constructs adaptive models.
- Pendulum with State-Space Representation: Generally, state-space representations must be employed for model analysis and management.
- Pendulum with Linear Matrix Inequalities: For control design, it is appreciable to implement linear matrix inequalities.
- Pendulum with Lyapunov-Based Control: The Lyapunov-based control policies should be applied.
- Pendulum with Time-Varying Parameters: On the movement of the pendulum, we explore the impacts of time-varying metrics.
- Pendulum with Hybrid Dynamics: The hybrid dynamics must be investigated which includes discrete and continuous models.
- Pendulum with Complex Dynamics: In the pendulum, we design complicated dynamics like disruptive characteristics.
- Pendulum with Adaptive Mesh Refinement: For precise simulations, it is advisable to utilize adaptive mesh refinement.
- Pendulum with Data-Driven Models: Mainly, for pendulum simulation and management, our team applies data-driven frameworks.
- Pendulum with Advanced Numerical Methods: For enhanced flexibility and precision, we investigate innovative numerical techniques.
Encompassing gradual procedures, instance code, and 100 project concepts, a detailed note on pendulum simulation is recommended by us which can be valuable for you in developing such kinds of projects.
We assist researchers in mastering Python Pendulum Simulation, providing all the essential tools and libraries. Reach out to matlabprojects.org for expert help and the best outcomes. Count on us for timely project delivery!
Subscribe Our Youtube Channel
You can Watch all Subjects Matlab & Simulink latest Innovative Project Results
Our services
We want to support Uncompromise Matlab service for all your Requirements Our Reseachers and Technical team keep update the technology for all subjects ,We assure We Meet out Your Needs.
Our Services
- Matlab Research Paper Help
- Matlab assignment help
- Matlab Project Help
- Matlab Homework Help
- Simulink assignment help
- Simulink Project Help
- Simulink Homework Help
- Matlab Research Paper Help
- NS3 Research Paper Help
- Omnet++ Research Paper Help
Our Benefits
- Customised Matlab Assignments
- Global Assignment Knowledge
- Best Assignment Writers
- Certified Matlab Trainers
- Experienced Matlab Developers
- Over 400k+ Satisfied Students
- Ontime support
- Best Price Guarantee
- Plagiarism Free Work
- Correct Citations
Expert Matlab services just 1-click
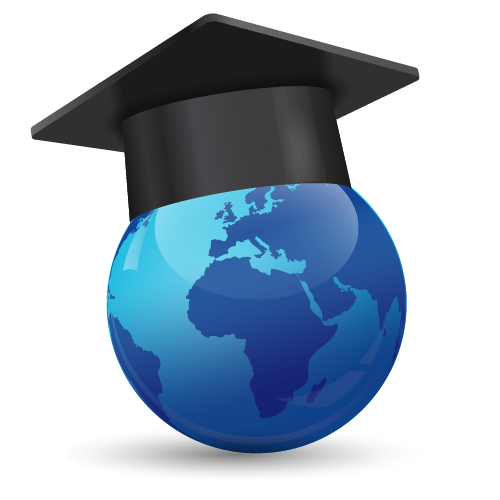
Delivery Materials
Unlimited support we offer you
For better understanding purpose we provide following Materials for all Kind of Research & Assignment & Homework service.
Programs
Designs
Simulations
Results
Graphs
Result snapshot
Video Tutorial
Instructions Profile
Sofware Install Guide
Execution Guidance
Explanations
Implement Plan
Matlab Projects
Matlab projects innovators has laid our steps in all dimension related to math works.Our concern support matlab projects for more than 10 years.Many Research scholars are benefited by our matlab projects service.We are trusted institution who supplies matlab projects for many universities and colleges.
Reasons to choose Matlab Projects .org???
Our Service are widely utilized by Research centers.More than 5000+ Projects & Thesis has been provided by us to Students & Research Scholars. All current mathworks software versions are being updated by us.
Our concern has provided the required solution for all the above mention technical problems required by clients with best Customer Support.
- Novel Idea
- Ontime Delivery
- Best Prices
- Unique Work
Simulation Projects Workflow

Embedded Projects Workflow
