Traffic Light Simulation in Python is one of the excellent ways to interpret state machines and how flow of traffic could be handled at a junction is the process of developing a traffic light simulation in Python. We suggest a basic instance of a traffic light simulation with Python. This instance will employ a state machine technique to handle traffic lights and the conversion between conditions:
Step 1: Install Required Libraries
Generally, we intend to employ matplotlib for visualizing the traffic lights and time for managing delays in this basic simulation.
pip install matplotlib
Step 2: Define the Traffic Light Simulation Code
import time
import matplotlib.pyplot as plt
import matplotlib.patches as patches
class TrafficLight:
def __init__(self):
self.state = “RED”
self.states = [“RED”, “GREEN”, “YELLOW”]
self.index = 0
self.durations = {“RED”: 5, “GREEN”: 5, “YELLOW”: 2} # Durations in seconds
def next_state(self):
self.index = (self.index + 1) % len(self.states)
self.state = self.states[self.index]
def get_duration(self):
return self.durations[self.state]
def draw_traffic_light(state):
fig, ax = plt.subplots()
ax.set_xlim(0, 10)
ax.set_ylim(0, 30)
# Draw the traffic light box
box = patches.Rectangle((3, 10), 4, 15, linewidth=1, edgecolor=’black’, facecolor=’grey’)
ax.add_patch(box)
# Draw the lights
red_light = patches.Circle((5, 23), 1.5, linewidth=1, edgecolor=’black’, facecolor=’red’ if state == “RED” else ‘darkred’)
green_light = patches.Circle((5, 15), 1.5, linewidth=1, edgecolor=’black’, facecolor=’green’ if state == “GREEN” else ‘darkgreen’)
yellow_light = patches.Circle((5, 19), 1.5, linewidth=1, edgecolor=’black’, facecolor=’yellow’ if state == “YELLOW” else ‘darkgoldenrod’)
ax.add_patch(red_light)
ax.add_patch(green_light)
ax.add_patch(yellow_light)
plt.axis(‘off’)
plt.show()
# Create a traffic light instance
traffic_light = TrafficLight()
# Run the simulation for a certain number of cycles
cycles = 5
for _ in range(cycles):
# Draw the current state of the traffic light
draw_traffic_light(traffic_light.state)
# Wait for the duration of the current state
time.sleep(traffic_light.get_duration())
# Transition to the next state
traffic_light.next_state()
Description of the Code
- TrafficLight Class:
- The condition of the traffic light such as GREEN, YELLOW, RED are handled in an appropriate manner.
- To obtain the time of the current condition, it includes suitable technique. Also encompasses a technique for conversion to the subsequent condition.
- draw_traffic_light Function:
- As a means to draw the traffic lights with circles depicting the lights, it is advisable to utilize matplotlib.
- On the basis of the existing condition, our team plans to color the lights.
- Main Simulation Loop:
- An instance of the TrafficLight class has to be constructed.
- In the meantime, for extracting the existing condition of traffic light, staying for the period of the condition, and conversions to the subsequent condition, we have to execute a loop for a fixed number of cycles.
traffic light simulation in python projects
There are numerous project ideas on traffic light simulation that are evolving continuously in recent years. We provide a collection of 100 project plans for traffic light simulation in Python:
Basic Simulations
- Two-Way Intersection Traffic Light
- Pedestrian Crossing Simulation
- Traffic Light with Countdown Timer
- Traffic Light Cycle Timing
- Flashing Yellow Light
- Single Intersection Traffic Light
- Four-Way Intersection Traffic Light
- Traffic Light with Timer Display
- Simple Traffic Light with Sensors
- Night Mode Traffic Light
Intermediate Simulations
- Adaptive Traffic Light Timing
- Traffic Light with Manual Override
- Traffic Light with Left Turn Signal
- Railroad Crossing Traffic Light
- Bus Rapid Transit Traffic Light
- Multi-Phase Traffic Light
- Traffic Light with Emergency Vehicle Override
- Traffic Light Synchronization
- Traffic Light with Right Turn Signal
- Roundabout Traffic Light
Advanced Simulations
- Intelligent Traffic Light System
- Traffic Light with Pedestrian Detection
- Traffic Light with Machine Learning for Optimization
- Traffic Light Control Using Reinforcement Learning
- Dynamic Traffic Light Control
- Simulating Traffic Light Network
- Traffic Light with Vehicle Detection
- Smart Traffic Light Using IoT
- Traffic Light with Real-Time Data Integration
- Simulating Traffic Congestion
Simulation with Visualization
- 3D Visualization of Traffic Light System
- Traffic Light Simulation with Pygame
- Web-Based Traffic Light Simulation
- Traffic Light Simulation with Vehicle Animation
- Traffic Light Simulation with VR
- 2D Visualization of Traffic Light System
- Animated Traffic Light Simulation
- Traffic Light Simulation with Tkinter
- Traffic Light Simulation with Real-Time Graphs
- Interactive Traffic Light Simulation
Multi-Intersection Simulations
- Traffic Light Synchronization Across Multiple Intersections
- Traffic Light Simulation for Large Cities
- Traffic Light Simulation for Suburban Areas
- Multi-Level Intersection Traffic Light Simulation
- Traffic Light Simulation with Flyovers
- Grid of Intersections
- Simulating Traffic Flow in Urban Areas
- Highway Traffic Light Simulation
- Traffic Light Simulation with Multiple Pedestrian Crossings
- Interconnected Traffic Light System
Real-World Data Integration
- Traffic Light Simulation with Weather Data Integration
- Traffic Light Simulation with Event Data Integration
- Traffic Light Simulation with Smart City Data
- Traffic Light Simulation with Mobile App Integration
- Traffic Light Simulation with Environmental Data
- Traffic Light Simulation with Real Traffic Data
- Traffic Light Simulation with Accident Data Integration
- Traffic Light Simulation Using GPS Data
- Traffic Light Simulation with Traffic Cameras
- Traffic Light Simulation with Public Transportation Data
Educational Simulations
- Traffic Light Simulation for Driver Education
- Traffic Light Simulation for Traffic Engineers
- Interactive Learning Tool for Traffic Management
- Traffic Light Simulation for Kids
- Traffic Light Simulation for Policy Makers
- Educational Tool for Traffic Light Timing
- Traffic Light Simulation for Pedestrian Safety Education
- Traffic Light Simulation for Urban Planning Students
- Traffic Light Simulation with Quiz Mode
- Traffic Light Behavior Simulation
Traffic Flow and Management
- Traffic Light Simulation for Traffic Flow Optimization
- Simulating Traffic Light Effects on Air Quality
- Simulating Traffic Light and Speed Limit Coordination
- Simulating Traffic Light and Toll Booth Integration
- Traffic Light Simulation for Special Events
- Simulating Traffic Light Impact on Traffic Flow
- Traffic Light Simulation for Reducing Traffic Jams
- Traffic Light Simulation for Emergency Evacuation
- Traffic Light Simulation for Peak and Off-Peak Hours
- Traffic Light Simulation for Freight Management
Innovative and Experimental Simulations
- Traffic Light Simulation with Autonomous Vehicles
- Simulating Future Traffic Light Technologies
- Blockchain-Based Traffic Light System
- Traffic Light Simulation for Space Colonies
- Traffic Light Simulation for Smart Roads
- Augmented Reality Traffic Light Simulation
- Traffic Light Simulation with Drone Integration
- Traffic Light Simulation with Energy Efficiency Focus
- Gamified Traffic Light Simulation
- Traffic Light Simulation with Biometric Detection
Research and Development
- Traffic Light Simulation for PhD Research
- Traffic Light Simulation for AI Research
- Traffic Light Simulation for Traffic Law Testing
- Traffic Light Simulation for Autonomous Traffic Management
- Traffic Light Simulation for Intelligent Transportation Systems
- Simulation of Traffic Light Algorithms
- Traffic Light Simulation for Traffic Signal Warrant Analysis
- Traffic Light Simulation for ITS Development
- Simulation of Traffic Light Impact on Urban Mobility
- Traffic Light Simulation for Future Urban Planning
We have offered a basic instance of a traffic light simulation with the aid of Python. Also, a collection of 100 project plans for traffic light simulations in Python are recommended by us in this article.
matlabprojects.org boasts a proficient team dedicated to Traffic Light Simulation in Python. We assure you of exceptional outcomes. Please share the details of your projects with our technical experts, as we possess all the necessary tools and are well-acquainted with the relevant libraries. We cover all aspects by offering you the finest project ideas and topics. Feel free to reach out with your requirements, and we will assist you in achieving optimal simulation results.
Subscribe Our Youtube Channel
You can Watch all Subjects Matlab & Simulink latest Innovative Project Results
Our services
We want to support Uncompromise Matlab service for all your Requirements Our Reseachers and Technical team keep update the technology for all subjects ,We assure We Meet out Your Needs.
Our Services
- Matlab Research Paper Help
- Matlab assignment help
- Matlab Project Help
- Matlab Homework Help
- Simulink assignment help
- Simulink Project Help
- Simulink Homework Help
- Matlab Research Paper Help
- NS3 Research Paper Help
- Omnet++ Research Paper Help
Our Benefits
- Customised Matlab Assignments
- Global Assignment Knowledge
- Best Assignment Writers
- Certified Matlab Trainers
- Experienced Matlab Developers
- Over 400k+ Satisfied Students
- Ontime support
- Best Price Guarantee
- Plagiarism Free Work
- Correct Citations
Expert Matlab services just 1-click
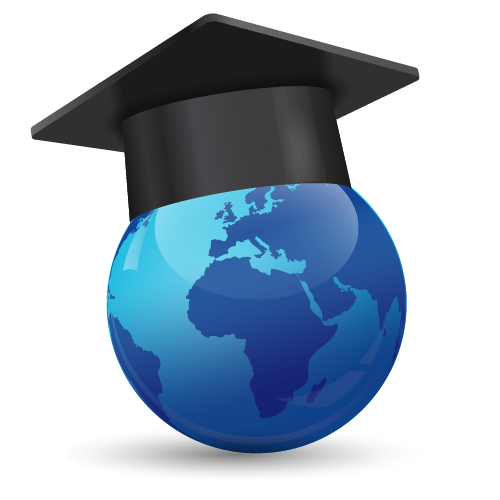
Delivery Materials
Unlimited support we offer you
For better understanding purpose we provide following Materials for all Kind of Research & Assignment & Homework service.
Programs
Designs
Simulations
Results
Graphs
Result snapshot
Video Tutorial
Instructions Profile
Sofware Install Guide
Execution Guidance
Explanations
Implement Plan
Matlab Projects
Matlab projects innovators has laid our steps in all dimension related to math works.Our concern support matlab projects for more than 10 years.Many Research scholars are benefited by our matlab projects service.We are trusted institution who supplies matlab projects for many universities and colleges.
Reasons to choose Matlab Projects .org???
Our Service are widely utilized by Research centers.More than 5000+ Projects & Thesis has been provided by us to Students & Research Scholars. All current mathworks software versions are being updated by us.
Our concern has provided the required solution for all the above mention technical problems required by clients with best Customer Support.
- Novel Idea
- Ontime Delivery
- Best Prices
- Unique Work
Simulation Projects Workflow

Embedded Projects Workflow
