CSE Projects Using Python are handled by our developers in a well appropriate way that suits individual requirements.Dont hesitate to approach matlabprojects.org experts we will give you good guidance and guide you with detailed implementation support. Python is one of the popular languages and is broadly used for developing web applications and effective platforms. Encompassing the dynamic programming, sorting, searching and graph algorithms, we provide several efficient algorithms along with suitable instance code:
Sorting Algorithms
- Bubble Sort
- Explanation: The bubble sort is a basic sorting method. The main process of this technique is that in a repetitive manner it treads across the list, compares neighbouring elements, and swaps them when they are in the illogical order.
- Python Code:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
arr = [64, 34, 25, 12, 22, 11, 90]
print(bubble_sort(arr))
- Selection Sort
- Explanation: Generally, the input list is splitted into two segments such as a sorted sublist of items and a sublist of the remaining unsorted items by a sorting method. From the unsorted sublist, it repetitively chooses the largest or smallest element and transfers it to the sorted sublist.
- Python Code:
def selection_sort(arr):
for i in range(len(arr)):
min_idx = i
for j in range(i+1, len(arr)):
if arr[j] < arr[min_idx]:
min_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
return arr
arr = [64, 25, 12, 22, 11]
print(selection_sort(arr))
- Insertion Sort
- Explanation: The final sorted array one item at one time is constructed by the insertion sort which is a basic sorting method. Compared to more innovative methods like merge sort, quicksort, or heapsort, it is much less effective on extensive lists.
- Python Code:
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i – 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
return arr
arr = [12, 11, 13, 5, 6]
print(insertion_sort(arr))
- Merge Sort
- Explanation: Merge sort is a divide and conquer method. In 1945, it was designed by John von Neumann. A consistent sort is generally offered by most of the executions, which means in a sorted output, the execution process maintains the input order of equivalent elements.
- Python Code:
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2
L = arr[:mid]
R = arr[mid:]
merge_sort(L)
merge_sort(R)
i = j = k = 0
while i < len(L) and j < len(R):
if L[i] < R[j]:
arr[k] = L[i]
i += 1
else:
arr[k] = R[j]
j += 1
k += 1
while i < len(L):
arr[k] = L[i]
i += 1
k += 1
while j < len(R):
arr[k] = R[j]
j += 1
k += 1
arr = [12, 11, 13, 5, 6, 7]
merge_sort(arr)
print(arr)
- Quick Sort
- Explanation: The quick sort is on the basis of division of an array of data into smaller arrays. It is examined as an extremely effective sorting method. Generally, an extensive array is divided into two arrays. Compared to the predefined values, one of the array maintains smaller values which is termed as pivot. In comparison with pivot value, the other array carries greater values as the result of which the splitting is developed.
- Python Code:
def partition(arr, low, high):
i = (low – 1)
pivot = arr[high]
for j in range(low, high):
if arr[j] <= pivot:
i = i + 1
arr[i], arr[j] = arr[j], arr[i]
arr[i + 1], arr[high] = arr[high], arr[i + 1]
return (i + 1)
def quick_sort(arr, low, high):
if low < high:
pi = partition(arr, low, high)
quick_sort(arr, low, pi – 1)
quick_sort(arr, pi + 1, high)
arr = [10, 7, 8, 9, 1, 5]
quick_sort(arr, 0, len(arr) – 1)
print(arr)
Searching Algorithms
- Linear Search
- Explanation: For identifying an element within a list, a linear search algorithm is employed. Till a match is identified or the entire list has been explored, it examines every element of the list in a sequential manner.
- Python Code:
def linear_search(arr, x):
for i in range(len(arr)):
if arr[i] == x:
return i
return -1
arr = [2, 3, 4, 10, 40]
x = 10
print(linear_search(arr, x))
- Binary Search
- Explanation: With a time intricacy of O(log n), the binary search is a fast search method. According to the principle of divide and conquer, this search method functions in an effective manner.
- Python Code:
def binary_search(arr, x):
low = 0
high = len(arr) – 1
mid = 0
while low <= high:
mid = (high + low) // 2
if arr[mid] < x:
low = mid + 1
elif arr[mid] > x:
high = mid – 1
else:
return mid
return -1
arr = [2, 3, 4, 10, 40]
x = 10
print(binary_search(arr, x))
Graph Algorithms
- Depth-First Search (DFS)
- Explanation: For searching or navigating tree or graph data structures, the DFS method is employed. At the root (in the event of a graph, choosing a certain random node as the root), the method initiates. Before backtracking, it discovers entirely across every branch.
- Python Code:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=’ ‘)
for next in graph[start] – visited:
dfs(graph, next, visited)
return visited
graph = {
‘A’: {‘B’, ‘C’},
‘B’: {‘A’, ‘D’, ‘E’},
‘C’: {‘A’, ‘F’},
‘D’: {‘B’},
‘E’: {‘B’, ‘F’},
‘F’: {‘C’, ‘E’}
}
dfs(graph, ‘A’)
- Breadth-First Search (BFS)
- Explanation: To search or navigate graph or tree data structures, BFS method is utilized. At the tree root or certain random node of a graph which is termed as ‘search key'[1], it begins. In advance of shifting on to the nodes at the subsequent depth phase, this algorithm examines the associated nodes at the current depth.
- Python Code:
def bfs(graph, start):
visited = []
queue = [start]
while queue:
node = queue.pop(0)
if node not in visited:
visited.append(node)
neighbours = graph[node]
for neighbour in neighbours:
queue.append(neighbour)
return visited
graph = {
‘A’: [‘B’, ‘C’],
‘B’: [‘D’, ‘E’],
‘C’: [‘F’],
‘D’: [],
‘E’: [‘F’],
‘F’: []
}
print(bfs(graph, ‘A’))
- Dijkstra’s Algorithm
- Explanation: In a graph, this algorithm is often used to detect the shortest routes among nodes. For instance, it can depict road networks.
- Python Code:
import sys
def dijkstra(graph, start):
shortest_paths = {start: (None, 0)}
current_node = start
visited = set()
while current_node is not None:
visited.add(current_node)
destinations = graph[current_node]
weight_to_current_node = shortest_paths[current_node][1]
for next_node, weight in destinations.items():
weight = weight_to_current_node + weight
if next_node not in shortest_paths:
shortest_paths[next_node] = (current_node, weight)
else:
current_shortest_weight = shortest_paths[next_node][1]
if current_shortest_weight > weight:
shortest_paths[next_node] = (current_node, weight)
next_destinations = {node: shortest_paths[node] for node in shortest_paths if node not in visited}
if not next_destinations:
return shortest_paths
current_node = min(next_destinations, key=lambda k: next_destinations[k][1])
graph = {
‘A’: {‘B’: 1, ‘C’: 4},
‘B’: {‘A’: 1, ‘C’: 2, ‘D’: 5},
‘C’: {‘A’: 4, ‘B’: 2, ‘D’: 1},
‘D’: {‘B’: 5, ‘C’: 1}
}
start = ‘A’
shortest_paths = dijkstra(graph, start)
print(shortest_paths)
Dynamic Programming Algorithms
- Fibonacci Sequence
- Explanation: Generally, Fibonacci sequence is the succession of numbers that begins with 0 and 1, in which every number is the total of the two previous numbers.
- Python Code:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
print(fibonacci(10))
- Longest Common Subsequence (LCS)
- Explanation: In a collection of sequences such as two sequences, the longest sequence shared by every sequence.
- Python Code:
def lcs(X, Y):
m = len(X)
n = len(Y)
L = [[None]*(n+1) for i in range(m+1)]
for i in range(m+1):
for j in range(n+1):
if i == 0 or j == 0:
L[i][j] = 0
elif X[i-1] == Y[j-1]:
L[i][j] = L[i-1][j-1] + 1
else:
L[i][j] = max(L[i-1][j], L[i][j-1])
return L[m][n]
X = “AGGTAB”
Y = “GXTXAYB”
print(“Length of LCS is”, lcs(X, Y))
- Knapsack Problem
- Explanation: Every item containing a value and a weight, a collection of items is specified. To encompass in a set, focus on defining the number of every item in such a manner the total value is as extensive as possible and the total weight is equivalent to or less than a specified constraint.
- Python Code:
def knapsack(W, wt, val, n):
K = [[0 for x in range(W + 1)] for x in range(n + 1)]
for i in range(n + 1):
for w in range(W + 1):
if i == 0 or w == 0:
K[i][w] = 0
elif wt[i-1] <= w:
K[i][w] = max(val[i-1] + K[i-1][w-wt[i-1]], K[i-1][w])
else:
K[i][w] = K[i-1][w]
return K[n][W]
val = [60, 100, 120]
wt = [10, 20, 30]
W = 50
n = len(val)
print(knapsack(W, wt, val, n))
String Algorithms
- Rabin-Karp Algorithm
- Explanation: In a text, identify a perfect match of a pattern string through applying string-searching method which employs hashing technique.
- Python Code:
def rabin_karp(text, pattern):
d = 256
q = 101
n = len(text)
m = len(pattern)
p = 0
t = 0
h = 1
for i in range(m-1):
h = (h*d) % q
for i in range(m):
p = (d*p + ord(pattern[i])) % q
t = (d*t + ord(text[i])) % q
for i in range(n-m+1):
if p == t:
for j in range(m):
if text[i+j] != pattern[j]:
break
j += 1
if j == m:
print(“Pattern found at index ” + str(i))
if i < n-m:
t = (d*(t-ord(text[i])*h) + ord(text[i+m])) % q
if t < 0:
t = t+q
text = “GEEKS FOR GEEKS”
pattern = “GEEK”
rabin_karp(text, pattern)
- Knuth-Morris-Pratt (KMP) Algorithm
- Explanation: Within a major text string, explore the existence of a word by a string-searching method. Through utilizing the investigation, the word itself represents adequate details when a mismatching happens, to define in which the initiation of the subsequent match occurs.
- Python Code:
def KMPSearch(pat, txt):
M = len(pat)
N = len(txt)
lps = [0]*M
j = 0
computeLPSArray(pat, M, lps)
i = 0
while i < N:
if pat[j] == txt[i]:
i += 1
j += 1
if j == M:
print(“Found pattern at index ” + str(i-j))
j = lps[j-1]
elif i < N and pat[j] != txt[i]:
if j != 0:
j = lps[j-1]
else:
i += 1
def computeLPSArray(pat, M, lps):
length = 0
lps[0] = 0
i = 1
while i < M:
if pat[i] == pat[length]:
length += 1
lps[i] = length
i += 1
else:
if length != 0:
length = lps[length-1]
else:
lps[i] = 0
i += 1
txt = “ABABDABACDABABCABAB”
pat = “ABABCABAB”
KMPSearch(pat, txt)
Computational Geometry Algorithms
- Convex Hull
- Explanation: The convex hull is capable of encompassing every provided point and is examined as the smallest convex points.
- Python Code:
from scipy.spatial import ConvexHull
import matplotlib.pyplot as plt
import numpy as np
points = np.random.rand(30, 2)
hull = ConvexHull(points)
plt.plot(points[:,0], points[:,1], ‘o’)
for simplex in hull.simplices:
plt.plot(points[simplex, 0], points[simplex, 1], ‘k-‘)
plt.show()
- Line Intersection
- Explanation: The line intersection algorithm is utilized to examine whether two provided line segments interconnect.
- Python Code:
def onSegment(p, q, r):
if (q[0] <= max(p[0], r[0]) and q[0] >= min(p[0], r[0]) and
q[1] <= max(p[1], r[1]) and q[1] >= min(p[1], r[1])):
return True
return False
def orientation(p, q, r):
val = (q[1] – p[1]) * (r[0] – q[0]) – (q[0] – p[0]) * (r[1] – q[1])
if val == 0:
return 0
return 1 if val > 0 else 2
def doIntersect(p1, q1, p2, q2):
o1 = orientation(p1, q1, p2)
o2 = orientation(p1, q1, q2)
o3 = orientation(p2, q2, p1)
o4 = orientation(p2, q2, q1)
if o1 != o2 and o3 != o4:
return True
if o1 == 0 and onSegment(p1, p2, q1):
return True
if o2 == 0 and onSegment(p1, q2, q1):
return True
if o3 == 0 and onSegment(p2, p1, q2):
return True
if o4 == 0 and onSegment(p2, q1, q2):
return True
return False
p1 = (1, 1)
q1 = (10, 1)
p2 = (1, 2)
q2 = (10, 2)
if doIntersect(p1, q1, p2, q2):
print(“Yes”)
else:
print(“No”)
Other Essential Algorithms
- Union-Find (Disjoint Set)
- Explanation: In the condition of segmented elements of various disjoint (not intersect) sets, this algorithm consistently monitors the elements.
- Python Code:
class DisjointSet:
def __init__(self, n):
self.parent = list(range(n))
self.rank = [0] * n
def find(self, u):
if self.parent[u] != u:
self.parent[u] = self.find(self.parent[u])
return self.parent[u]
def union(self, u, v):
root_u = self.find(u)
root_v = self.find(v)
if root_u != root_v:
if self.rank[root_u] > self.rank[root_v]:
self.parent[root_v] = root_u
else:
self.parent[root_u] = root_v
if self.rank[root_u] == self.rank[root_v]:
self.rank[root_v] += 1
ds = DisjointSet(5)
ds.union(0, 2)
ds.union(4, 2)
ds.union(3, 1)
print(ds.find(4))
print(ds.find(3))
- Topological Sort
- Explanation: Typically, topological sort is a linear ordering of vertices of a Directed Acyclic Graph (DAG). In the arrangement, for each directed edge uv, vertex v comes after u.
- Python Code:
from collections import defaultdict
class Graph:
def __init__(self, vertices):
self.graph = defaultdict(list)
self.V = vertices
def add_edge(self, u, v):
self.graph[u].append(v)
def topological_sort_util(self, v, visited, stack):
visited[v] = True
for i in self.graph[v]:
if not visited[i]:
self.topological_sort_util(i, visited, stack)
stack.insert(0, v)
def topological_sort(self):
visited = [False] * self.V
stack = []
for i in range(self.V):
if not visited[i]:
self.topological_sort_util(i, visited, stack)
print(stack)
g = Graph(6)
g.add_edge(5, 2)
g.add_edge(5, 0)
g.add_edge(4, 0)
g.add_edge(4, 1)
g.add_edge(2, 3)
g.add_edge(3, 1)
g.topological_sort()
- Bellman-Ford Algorithm
- Explanation: In a weighted digraph, Bellman-Ford method is employed to identify the shortest routes from a single source vertex to every other vertex.
- Python Code:
def bellman_ford(graph, V, E, src):
dist = [float(“Inf”)] * V
dist[src] = 0
for i in range(V – 1):
for u, v, w in E:
if dist[u] != float(“Inf”) and dist[u] + w < dist[v]:
dist[v] = dist[u] + w
for u, v, w in E:
if dist[u] != float(“Inf”) and dist[u] + w < dist[v]:
print(“Graph contains negative weight cycle”)
return
print(“Vertex Distance from Source”)
for i in range(V):
print(“{0}\t\t{1}”.format(i, dist[i]))
V = 5
E = 8
edges = [(0, 1, -1), (0, 2, 4), (1, 2, 3), (1, 3, 2), (1, 4, 2), (3, 2, 5), (3, 1, 1), (4, 3, -3)]
bellman_ford(edges, V, E, 0)
Implementations of Advanced Algorithms
- A Search Algorithm*
- Explanation: Mainly, A* search is considered as a graph traversal and pathfinding method. As a result of its improved effectiveness, extensiveness, and efficiency, it is commonly utilized in numerous domains of computer science.
- Python Code:
from queue import PriorityQueue
def a_star_search(graph, start, goal):
open_list = PriorityQueue()
open_list.put((0, start))
came_from = {start: None}
cost_so_far = {start: 0}
while not open_list.empty():
current = open_list.get()[1]
if current == goal:
break
for neighbor in graph[current]:
new_cost = cost_so_far[current] + graph[current][neighbor]
if neighbor not in cost_so_far or new_cost < cost_so_far[neighbor]:
cost_so_far[neighbor] = new_cost
priority = new_cost + heuristic(goal, neighbor)
open_list.put((priority, neighbor))
came_from[neighbor] = current
return came_from, cost_so_far
def heuristic(a, b):
(x1, y1) = a
(x2, y2) = b
return abs(x1 – x2) + abs(y1 – y2)
graph = {
‘A’: {‘B’: 1, ‘C’: 4},
‘B’: {‘A’: 1, ‘C’: 2, ‘D’: 5},
‘C’: {‘A’: 4, ‘B’: 2, ‘D’: 1},
‘D’: {‘B’: 5, ‘C’: 1}
}
start = ‘A’
goal = ‘D’
came_from, cost_so_far = a_star_search(graph, start, goal)
print(“Path:”, came_from)
print(“Cost:”, cost_so_far)
- Kruskal’s Algorithm
- Explanation: Typically, Kruskal’s algorithm is examined as the graph theory method. For a connected weighted graph, identify a minimum spanning tree through employing this algorithm.
- Python Code:
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = []
def add_edge(self, u, v, w):
self.graph.append([u, v, w])
def find(self, parent, i):
if parent[i] == i:
return i
return self.find(parent, parent[i])
def union(self, parent, rank, x, y):
root_x = self.find(parent, x)
root_y = self.find(parent, y)
if rank[root_x] < rank[root_y]:
parent[root_x] = root_y
elif rank[root_x] > rank[root_y]:
parent[root_y] = root_x
else:
parent[root_y] = root_x
rank[root_x] += 1
def kruskal(self):
result = []
i = 0
e = 0
self.graph = sorted(self.graph, key=lambda item: item[2])
parent = []
rank = []
for node in range(self.V):
parent.append(node)
rank.append(0)
while e < self.V – 1:
u, v, w = self.graph[i]
i = i + 1
x = self.find(parent, u)
y = self.find(parent, v)
if x != y:
e = e + 1
result.append([u, v, w])
self.union(parent, rank, x, y)
print(“Following are the edges in the constructed MST”)
for u, v, weight in result:
print(f”{u} — {v} == {weight}”)
g = Graph(4)
g.add_edge(0, 1, 10)
g.add_edge(0, 2, 6)
g.add_edge(0, 3, 5)
g.add_edge(1, 3, 15)
g.add_edge(2, 3, 4)
g.kruskal()
Practical Use-Case Algorithms
- PageRank Algorithm
- Explanation: In order to rank web pages in the search engine outcomes, this technique is utilized by Google Search.
- Python Code:
import numpy as np
def pagerank(M, num_iterations: int = 100, d: float = 0.85):
N = M.shape[1]
v = np.random.rand(N, 1)
v = v / np.linalg.norm(v, 1)
M_hat = (d * M + (1 – d) / N)
for _ in range(num_iterations):
v = M_hat @ v
return v
M = np.array([[0, 0, 1, 0],
[1/2, 0, 0, 1/2],
[1/2, 1, 0, 1/2],
[0, 0, 0, 0]])
print(pagerank(M, 100, 0.85))
- Apriori Algorithm
- Explanation: For extracting regular itemsets and learning related association regulations, the Apriori method is employed.
- Python Code:
def apriori(transactions, min_support):
itemset = set()
for transaction in transactions:
for item in transaction:
itemset.add(frozenset([item]))
freq_itemset = dict()
k = 1
current_itemset = {i for i in itemset if support(transactions, i) >= min_support}
while current_itemset:
freq_itemset.update({item: support(transactions, item) for item in current_itemset})
current_itemset = set([i.union(j) for i in current_itemset for j in current_itemset if len(i.union(j)) == k + 1])
current_itemset = {i for i in current_itemset if support(transactions, i) >= min_support}
k += 1
return freq_itemset
def support(transactions, itemset):
return sum(1 for transaction in transactions if itemset.issubset(transaction)) / len(transactions)
transactions = [
{‘bread’, ‘milk’},
{‘bread’, ‘diaper’, ‘beer’, ‘egg’},
{‘milk’, ‘diaper’, ‘beer’, ‘cola’},
{‘bread’, ‘milk’, ‘diaper’, ‘beer’},
{‘bread’, ‘milk’, ‘diaper’, ‘cola’},
]
print(apriori(transactions, 0.6))
cse python projects list
There exist numerous Python projects, but some are determined as fascinating and significant. Together with concise outlines, we recommend 10 captivating Python projects for CSE students. These plans could motivate you and assist you to investigate various regions of computer science:
- Chatbot using Natural Language Processing (NLP)
- Outline: To interpret user questions and answer in an appropriate manner, we plan to construct an efficient chatbot. In order to process natural language and create answers, it is beneficial to employ NLP libraries of Python such as transformers, nltk, and spaCy.
- Major Concepts: Text Processing, Sentiment Analysis, NLP, Machine Learning.
- Face Detection and Recognition System
- Outline: Through the utilization of dlib and OpenCV libraries of Python, identify and classify faces by developing a framework. With the aid of deep learning methods, our team focuses on applying face recognition approaches.
- Major Concepts: Deep Learning, Image Processing, OpenCV, Neural Networks.
- AI-based Stock Price Predictor
- Outline: On the basis of past data, we intend to forecast stock prices by means of employing machine learning methods like ARIMA or LSTM (Long Short-Term Memory). For data analysis and modeling, it is significant to utilize TensorFlow, pandas, and scikit-learn.
- Major Concepts: Neural Networks, Time Series Analysis, Machine Learning.
- Recommendation System (Movie/Book Recommender)
- Outline: With the support of content-based filtering or collaborative filtering, we aim to develop a recommendation engine for books or movies. Focus on employing Python libraries such as surprise, pandas, and scikit-learn.
- Major Concepts: Machine Learning, Recommendation Algorithms, Data Science.
- Personal Finance Management System
- Outline: To assist users to handle their savings, income, and expenditures, our team plans to construct a Python-based finance management framework. For the user interface, it is beneficial to employ Flask or Tkinter.
- Major Concepts: Financial Analysis, Python GUI, Data Management.
- Social Media Sentiment Analysis
- Outline: For scrabbling social media data like (from Twitter), we develop an effective tool and after every post, evaluate the sentiment. Focus on employing nltk for sentiment analysis and Tweepy for data gathering.
- Major Concepts: Sentiment Analysis, Data Visualization, Web Scraping, NLP.
- Python File Encryption System
- Outline: By means of employing cryptographic approaches like RSA or AES, protect confidential data through creating a file encryption and decryption model.
- Major Concepts: Encryption Algorithms, Cryptography, Security.
- Handwritten Digit Recognition (MNIST)
- Outline: Through the utilization of the MNIST dataset, identify handwritten digits by instructing a neural network. As a means to execute deep learning systems such as CNN (Convolutional Neural Networks), it is advisable to employ PyTorch or TensorFlow.
- Major Concepts: Image Processing, Deep Learning, Convolutional Neural Networks.
- Web Scraping Tool
- Outline: To obtain beneficial data from websites and save it in a CSV file or database, our team intends to develop a web scraping tool. It is appreciable to utilize Scrapy, BeautifulSoup, and Selenium of Python.
- Major Concepts: Automation, Web Scraping, Data Extraction.
- Flask-based Blog Website
- Outline: With the aid of Flask, a Python-based micro-framework, we plan to construct a blog website. Generally, significant characteristics such as commenting models, user authentication, and blog creation should be appended.
- Major Concepts: Databases (SQL/NoSQL), Web Development, Flask.
There are several CSE Python project ideas progressing continuously in recent years. We offer a collection of 100 CSE Python project plans among different fields like web development, data science, automation, machine learning, networking, cybersecurity, and more:
- Web Development
- E-commerce Website with Django
- Social Media App using Django
- Personal Finance Management System
- News Aggregator Website using Flask
- Event Management System using Django
- Blog Website using Flask or Django
- Portfolio Website using Flask
- To-Do List Application with Flask
- Job Portal Website using Django
- Real-Time Chat Application with Flask and Socket.io
- Machine Learning
- Image Classification using CNN (Convolutional Neural Networks)
- Stock Price Prediction using LSTM
- Breast Cancer Prediction using Machine Learning
- Sentiment Analysis on Social Media Posts
- Face Detection and Recognition using OpenCV and Deep Learning
- Handwritten Digit Recognition (MNIST) using TensorFlow
- Movie Recommendation System using Collaborative Filtering
- Spam Email Detection using NLP
- House Price Prediction using Linear Regression
- Music Genre Classification using KNN
- Data Science
- Sales Data Analysis using Pandas
- Exploratory Data Analysis on Titanic Dataset
- Twitter Sentiment Analysis using Tweepy
- Uber Data Analysis with Python
- Crime Data Analysis using Pandas and Seaborn
- Weather Forecasting using Machine Learning
- Data Cleaning Tool using Pandas and Numpy
- Fake News Detection using NLP
- Customer Segmentation using K-Means Clustering
- COVID-19 Data Analysis and Visualization
- Cybersecurity
- Network Packet Sniffer using Scapy
- Keylogger Detection System
- Malware Analysis Automation Tool
- Port Scanner using Python and Socket
- Intrusion Detection System using Machine Learning
- Password Strength Checker using Python
- File Encryption and Decryption System using AES
- Web Vulnerability Scanner using Python
- DDoS Attack Simulation using Python
- Firewall Simulation in Python
- Networking
- Simple Web Server using Python
- FTP Client and Server in Python
- Remote Desktop Access Tool
- Email Client using Python’s SMTP and IMAP
- IP Geolocation Finder using APIs
- Socket Programming Chat Application
- Network Bandwidth Monitor
- TCP Packet Sniffing Tool
- File Transfer System using Sockets
- Network Traffic Analyzer using Scapy
- Automation
- Automated Resume Builder
- Instagram Bot for Auto-Liking and Commenting
- Bulk Email Sender using SMTP
- WhatsApp Message Sender Bot
- File Organizer Automation
- Web Scraping using BeautifulSoup and Selenium
- PDF to Audio Converter using PyPDF2
- YouTube Video Downloader using Pytube
- Automated Attendance System with Face Recognition
- Voice Assistant using Speech Recognition
- Game Development
- Snake Game using Pygame
- Sudoku Solver and Generator using Backtracking
- Flappy Bird Clone using Pygame
- Minesweeper Game in Python
- Space Shooter Game using Pygame
- Tic-Tac-Toe Game in Python
- Hangman Game in Python
- Chess Game using Python
- Car Racing Game using Pygame
- Breakout Game using Pygame
- Artificial Intelligence
- Self-Driving Car Simulation
- AI-Based Chess Game Solver
- Traffic Sign Recognition System using Deep Learning
- AI-Based Resume Screening Tool
- Automated Essay Grading System using NLP
- Chatbot using NLP (Natural Language Processing)
- AI Virtual Assistant using Python
- Image Caption Generator using CNN and LSTM
- AI-Driven Personalized Learning System
- Human Pose Detection using OpenCV
- Cloud Computing
- Cloud File Storage System using Python
- Virtual Machine Management using Python (libcloud)
- Cloud-Based Video Streaming Application
- Serverless Application with Google Cloud Functions
- AWS EC2 Instance Auto-Scaling Script
- Deploy a Machine Learning Model on AWS using Flask
- Building a Serverless API with AWS Lambda and Python
- Cloud-based Data Backup System
- AWS S3 File Uploader using Python
- Web App Deployment with Docker and Python
- Miscellaneous
- Barcode and QR Code Generator
- Plagiarism Detection Tool using NLP
- Sudoku Solver using Backtracking Algorithm
- Music Player Application in Python
- GUI Calculator using Tkinter
- Personal Expense Tracker with SQLite
- Online Quiz System with Python and MySQL
- Text to Speech Converter using gTTS
- AI Chatbot for Healthcare Support
- Weather Application using APIs
Through this article, we have suggested numerous methods that include a broad scope of topics such as searching, dynamic programming, sorting, graph methods, and more. Encompassing short outlines, 10 intriguing Python projects for CSE students and a collection of 100 CSE Python project plans among several disciplines like web development, data science, automation, machine learning, networking, cybersecurity are suggested by us in an explicit manner.
Subscribe Our Youtube Channel
You can Watch all Subjects Matlab & Simulink latest Innovative Project Results
Our services
We want to support Uncompromise Matlab service for all your Requirements Our Reseachers and Technical team keep update the technology for all subjects ,We assure We Meet out Your Needs.
Our Services
- Matlab Research Paper Help
- Matlab assignment help
- Matlab Project Help
- Matlab Homework Help
- Simulink assignment help
- Simulink Project Help
- Simulink Homework Help
- Matlab Research Paper Help
- NS3 Research Paper Help
- Omnet++ Research Paper Help
Our Benefits
- Customised Matlab Assignments
- Global Assignment Knowledge
- Best Assignment Writers
- Certified Matlab Trainers
- Experienced Matlab Developers
- Over 400k+ Satisfied Students
- Ontime support
- Best Price Guarantee
- Plagiarism Free Work
- Correct Citations
Expert Matlab services just 1-click
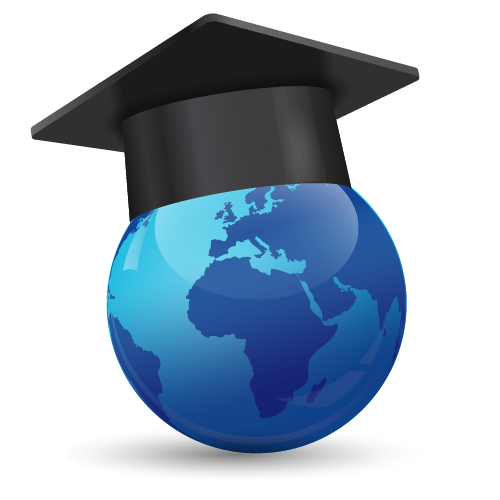
Delivery Materials
Unlimited support we offer you
For better understanding purpose we provide following Materials for all Kind of Research & Assignment & Homework service.
Programs
Designs
Simulations
Results
Graphs
Result snapshot
Video Tutorial
Instructions Profile
Sofware Install Guide
Execution Guidance
Explanations
Implement Plan
Matlab Projects
Matlab projects innovators has laid our steps in all dimension related to math works.Our concern support matlab projects for more than 10 years.Many Research scholars are benefited by our matlab projects service.We are trusted institution who supplies matlab projects for many universities and colleges.
Reasons to choose Matlab Projects .org???
Our Service are widely utilized by Research centers.More than 5000+ Projects & Thesis has been provided by us to Students & Research Scholars. All current mathworks software versions are being updated by us.
Our concern has provided the required solution for all the above mention technical problems required by clients with best Customer Support.
- Novel Idea
- Ontime Delivery
- Best Prices
- Unique Work
Simulation Projects Workflow

Embedded Projects Workflow
