Fluid Simulation in Python is the process of simulating 2D fluid is determined as challenging as well as fascinating. Generally, derived from the Stable Fluids technique by Jos Stam, we exhibit a simple method to 2D fluid simulation with the aid of Numpy and Python. If you encounter any challenges, please feel free to communicate your research needs to matlabprojects.org. We possess the most up-to-date resources and a dedicated technical team ready to assist you in achieving high-quality results efficiently. We carry on thesis writing in an effective manner that it will be nil from plagiarism. This technique is considered as an excellent beginning for interpreting fluid dynamics simulations:
Basic 2D Fluid Simulation Using Python and NumPy
Procedural Explanation
- Initialization: Initially, for density, velocity (u, v), and external forces, we plan to develop grids.
- Advection: Across the grid, our team aims to transfer quantities such as density and velocity.
- Diffusion: Generally, across the grid the quantities have to be diffused.
- Projection: In a divergence-free (incompressible) manner, focus on creating the velocity field.
- External Forces: On the fluid like gravity, we aim to append any external impacts.
- Time Stepping: As a means to simulate the motion of the fluid, it is appreciable to repeat periodically.
Implementation
The following is a basic execution in Python:
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
# Simulation parameters
N = 64
size = (N + 2, N + 2)
dt = 0.1
diffusion = 0.0001
viscosity = 0.0001
# Initialize velocity, density, and force fields
u = np.zeros(size)
v = np.zeros(size)
u_prev = np.zeros(size)
v_prev = np.zeros(size)
density = np.zeros(size)
density_prev = np.zeros(size)
def add_source(x, s, dt):
x += dt * s
def diffuse(b, x, x0, diff, dt):
a = dt * diff * N * N
for _ in range(20):
x[1:-1, 1:-1] = (x0[1:-1, 1:-1] + a * (x[2:, 1:-1] + x[:-2, 1:-1] +
x[1:-1, 2:] + x[1:-1, :-2])) / (1 + 4 * a)
set_bnd(b, x)
def advect(b, d, d0, u, v, dt):
Nfloat = float(N)
dt0 = dt * N
for i in range(1, N + 1):
for j in range(1, N + 1):
x = i – dt0 * u[i, j]
y = j – dt0 * v[i, j]
if x < 0.5: x = 0.5
if x > N + 0.5: x = N + 0.5
if y < 0.5: y = 0.5
if y > N + 0.5: y = N + 0.5
i0 = int(x)
i1 = i0 + 1
j0 = int(y)
j1 = j0 + 1
s1 = x – i0
s0 = 1 – s1
t1 = y – j0
t0 = 1 – t1
d[i, j] = s0 * (t0 * d0[i0, j0] + t1 * d0[i0, j1]) + s1 * (t0 * d0[i1, j0] + t1 * d0[i1, j1])
set_bnd(b, d)
def project(u, v, p, div):
h = 1.0 / N
div[1:-1, 1:-1] = -0.5 * h * (u[2:, 1:-1] – u[:-2, 1:-1] + v[1:-1, 2:] – v[1:-1, :-2])
p[1:-1, 1:-1] = 0
set_bnd(0, div)
set_bnd(0, p)
for _ in range(20):
p[1:-1, 1:-1] = (div[1:-1, 1:-1] + p[2:, 1:-1] + p[:-2, 1:-1] +
p[1:-1, 2:] + p[1:-1, :-2]) / 4
set_bnd(0, p)
u[1:-1, 1:-1] -= 0.5 * (p[2:, 1:-1] – p[:-2, 1:-1]) / h
v[1:-1, 1:-1] -= 0.5 * (p[1:-1, 2:] – p[1:-1, :-2]) / h
set_bnd(1, u)
set_bnd(2, v)
def set_bnd(b, x):
x[0, :] = x[1, :] if b != 1 else -x[1, :]
x[-1, :] = x[-2, :] if b != 1 else -x[-2, :]
x[:, 0] = x[:, 1] if b != 2 else -x[:, 1]
x[:, -1] = x[:, -2] if b != 2 else -x[:, -2]
x[0, 0] = 0.5 * (x[1, 0] + x[0, 1])
x[0, -1] = 0.5 * (x[1, -1] + x[0, -2])
x[-1, 0] = 0.5 * (x[-2, 0] + x[-1, 1])
x[-1, -1] = 0.5 * (x[-2, -1] + x[-1, -2])
def vel_step(u, v, u0, v0, visc, dt):
add_source(u, u0, dt)
add_source(v, v0, dt)
u0, u = u, u0
v0, v = v, v0
diffuse(1, u, u0, visc, dt)
diffuse(2, v, v0, visc, dt)
project(u, v, u0, v0)
u0, u = u, u0
v0, v = v, v0
advect(1, u, u0, u0, v0, dt)
advect(2, v, v0, u0, v0, dt)
project(u, v, u0, v0)
def dens_step(x, x0, u, v, diff, dt):
add_source(x, x0, dt)
x0, x = x, x0
diffuse(0, x, x0, diff, dt)
x0, x = x, x0
advect(0, x, x0, u, v, dt)
# Animation setup
fig, ax = plt.subplots()
img = ax.imshow(density, interpolation=’bilinear’, cmap=’viridis’, origin=’lower’)
ax.set_title(‘2D Fluid Simulation’)
plt.colorbar(img, ax=ax)
def update(frame):
global u, v, u_prev, v_prev, density, density_prev
dens_step(density, density_prev, u, v, diffusion, dt)
vel_step(u, v, u_prev, v_prev, viscosity, dt)
density_prev.fill(0)
u_prev.fill(0)
v_prev.fill(0)
img.set_array(density)
return [img]
ani = animation.FuncAnimation(fig, update, frames=200, interval=50, blit=True)
plt.show()
Description
- Initialization: We focus on setting the density field and the velocity fields u and v to zeros. The grid size is described by the size variable.
- Functions:
- add_source: To the fields, this function includes source terms.
- diffuse: It is capable of distributing quantities such as density and velocity.
- advect: By means of velocity fields, it transfers quantities across the grid.
- project: This function makes sure that the velocity field is incompressible.
- set_bnd: Typically, it initializes the limit constraints.
- vel_step and dens_step: In density and velocity fields, it carries out a step in a proper manner.
- Animation: As a means to visualize the simulation, focus on employing Matplotlib.
Important 50 fluid simulation python Project Topics
In the motive of assisting you in selecting crucial and impactful fluid simulation project topics, few of the effective and significant project topics using Python are offered by us. These projects extend from simple fluid dynamics simulations to more complicated and implemented settings:
Basic Fluid Dynamics
- 2D Smoke Simulation
- Explanation: Through the utilization of the Navier-Stokes equations, we focus on simulating the motion and dispersion of smoke in a 2D grid.
- 2D Water Simulation
- Explanation: For indicating fluid communication and wave propagation, our team aims to develop a basic 2D water simulation.
- Particle-Based Fluid Simulation
- Explanation: With the support of the particle-based technique like Smoothed Particle Hydrodynamics (SPH), it is appreciable to execute a fluid simulation.
- Navier-Stokes Solver
- Explanation: Considering the Navier-Stokes equations in 2D, a solver must be created for steady-state flow.
- Lattice Boltzmann Method
- Explanation: Specifically, for simulating flow of fluid in 2D, we intend to apply the Lattice Boltzmann Method (LBM).
- Shallow Water Equations
- Explanation: As a means to simulate propagating wave and shallow water flows, our team plans to resolve the shallow water equations.
- Eulerian Fluid Simulation
- Explanation: For pressure and velocity fields, it is significant to develop an Eulerian fluid simulation with the support of a fixed grid technique.
- Vortex Method
- Explanation: With the aid of the vortex technique, we aim to simulate the flow of a fluid. In order to depict the movement of the fluid, focus on monitoring vortex particles.
- Rayleigh-Taylor Instability
- Explanation: The Rayleigh-Taylor instability must be simulated in which heavier fluid forces into a lowest density fluid under the influence of gravitational force.
- Kelvin-Helmholtz Instability
- Explanation: Typically, the Kelvin-Helmholtz instability has to be simulated. In the communication among two fluids with various velocities, this variability exists.
Advanced Fluid Dynamics
- 3D Smoke Simulation
- Explanation: By simulating the motion and dispersion of smoke in a 3D grid, we intend to prolong 2D smoke simulation to 3D.
- 3D Water Simulation
- Explanation: Encompassing wave propagation and surface tension, it is approachable to simulate 3D flow of water.
- SPH for 3D Fluids
- Explanation: For simulating 3D fluid flow, our team focuses on applying Smoothed Particle Hydrodynamics (SPH).
- Incompressible SPH
- Explanation: In order to simulate fluids with consistent density, we aim to construct an incompressible SPH solver.
- Fluid-Structure Interaction
- Explanation: Through the utilization of a coupled solver, it is advisable to simulate the communication among solid architectures and fluid flow.
- Multiphase Flow
- Explanation: Typically, multiphase flow encompassing two or more incompatible fluids must be simulated.
- Turbulence Modeling
- Explanation: We plan to apply turbulence systems like Direct Numerical Simulation (DNS), or Large Eddy Simulation (LES).
- Thermal Fluids
- Explanation: By resolving the coupled Navier-Stokes and heat equations, our team aims to simulate flow of a fluid with heat transfer.
- Cavitation Simulation
- Explanation: In the case of local low pressures, we need to simulate the cavitation event in which vapor bubbles form in a liquid.
- Non-Newtonian Fluids
- Explanation: Generally, non-Newtonian fluids ought to be simulated in which density varies on the frequency of shear strain.
Applications and Specialized Topics
- Fluid Flow in Pipes
- Explanation: Involving laminar and turbulent flow regimes, flow of a fluid across pipes must be simulated.
- Aerodynamics of Airfoils
- Explanation: To investigate drag and lift forces, we plan to simulate the flow of air across an airfoil.
- Dam Break Simulation
- Explanation: Through the utilization of fluid dynamics strategies, our team simulates the unexpected release of water from a dam.
- Ocean Wave Simulation
- Explanation: The distribution of ocean waves should be designed and simulated.
- River Flow Simulation
- Explanation: Encompassing communications with the riverbed and banks, it is appreciable to simulate the flow of a river.
- Sediment Transport
- Explanation: In a flowing fluid, we plan to simulate the transportation of sediment.
- Blood Flow in Arteries
- Explanation: Focusing on vessel flexibility and pulse rate, our team intends to design and simulate blood flow across arteries.
- Atmospheric Fluid Dynamics
- Explanation: Generally, extensive atmospheric events like weather trends and cloud architecture have to be simulated.
- Wind Tunnel Simulation
- Explanation: To simulate and examine flow of air across objects, we focus on developing an effective wind tunnel.
- Oil Spill Simulation
- Explanation: In an aquatic environment, the diffusion and distribution of oil spills should be designed.
Visualization and Interactive Simulations
- Real-Time Fluid Simulation
- Explanation: For interactive applications, our team aims to construct a real-time fluid simulation.
- Interactive Smoke Simulation
- Explanation: An interactive 2D smoke simulation has to be developed in which users are capable of guiding the movement of the flow.
- Augmented Reality Fluid Simulation
- Explanation: For visualizing fluid flows in actual world platforms, we focus on combining fluid simulations with augmented reality.
- Virtual Reality Fluid Simulation
- Explanation: To engage users in a simulated fluid platform, it is advisable to construct a VR application.
- Fluid Simulation for Games
- Explanation: Appropriate for actual time applications in video games, our team plans to apply fluid simulation methods.
- Data-Driven Fluid Simulation
- Explanation: In order to speed up and improve fluid simulations, we intend to employ machine learning approaches.
- GPU-Accelerated Fluid Simulation
- Explanation: To accelerate fluid simulations with models such as OpenCL or CUDA, our team plans to make use of GPU computing.
- Interactive Educational Tools
- Explanation: By means of employing interactive simulations, instruct fluid dynamics theories in an explicit manner through developing effective educational tools.
- Artistic Fluid Simulation
- Explanation: As a means to construct visually attractive special effects for digital art and animations, it is beneficial to utilize fluid simulations.
- Fluid Simulation for Special Effects
- Explanation: For developing practicable specific effects in animations and movies, our team focuses on executing fluid simulation.
Fluid Simulation in Engineering and Science
- Microfluidics Simulation
- Explanation: In micro-scale devices for lab-on-a-chip applications, flow of the fluid has to be simulated.
- Combustion Simulation
- Explanation: Encompassing heat transfer and flame distribution, we intend to design the fluid dynamics of combustion procedures.
- Chemical Mixing Simulation
- Explanation: In a flow of a fluid, our team aims to simulate the combination of various chemicals.
- HVAC System Simulation
- Explanation: Typically, in heating, ventilation, and air conditioning models, it is appreciable to simulate flow of air and temperature dissemination.
- Groundwater Flow Simulation
- Explanation: Across the porous medium, our team designs the flow of groundwater.
- Pollutant Dispersion in Air
- Explanation: In the atmosphere, we plan to simulate the diffusion of contaminants.
- Rainwater Harvesting Simulation
- Explanation: In harvesting models, our team aims to design the aggregation and flow of rainwater.
- Wave Energy Simulation
- Explanation: The communication of ocean waves with wave energy converters has to be simulated.
- Flood Modeling
- Explanation: Generally, urban and river flooding settings and their influences ought to be simulated.
- Fluid Flow in Porous Media
- Explanation: Appropriate for oil recovery and groundwater investigation, we simulate the flow of the fluid across porous resources.
Including procedural description, implementation, concise outline, and 50 important project concepts, an extensive note on 2D fluid simulation is recommended by us which can be valuable for you in developing such kinds of projects.
Subscribe Our Youtube Channel
You can Watch all Subjects Matlab & Simulink latest Innovative Project Results
Our services
We want to support Uncompromise Matlab service for all your Requirements Our Reseachers and Technical team keep update the technology for all subjects ,We assure We Meet out Your Needs.
Our Services
- Matlab Research Paper Help
- Matlab assignment help
- Matlab Project Help
- Matlab Homework Help
- Simulink assignment help
- Simulink Project Help
- Simulink Homework Help
- Matlab Research Paper Help
- NS3 Research Paper Help
- Omnet++ Research Paper Help
Our Benefits
- Customised Matlab Assignments
- Global Assignment Knowledge
- Best Assignment Writers
- Certified Matlab Trainers
- Experienced Matlab Developers
- Over 400k+ Satisfied Students
- Ontime support
- Best Price Guarantee
- Plagiarism Free Work
- Correct Citations
Expert Matlab services just 1-click
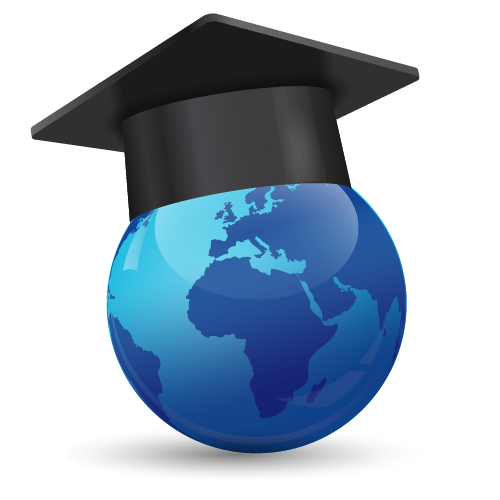
Delivery Materials
Unlimited support we offer you
For better understanding purpose we provide following Materials for all Kind of Research & Assignment & Homework service.
Programs
Designs
Simulations
Results
Graphs
Result snapshot
Video Tutorial
Instructions Profile
Sofware Install Guide
Execution Guidance
Explanations
Implement Plan
Matlab Projects
Matlab projects innovators has laid our steps in all dimension related to math works.Our concern support matlab projects for more than 10 years.Many Research scholars are benefited by our matlab projects service.We are trusted institution who supplies matlab projects for many universities and colleges.
Reasons to choose Matlab Projects .org???
Our Service are widely utilized by Research centers.More than 5000+ Projects & Thesis has been provided by us to Students & Research Scholars. All current mathworks software versions are being updated by us.
Our concern has provided the required solution for all the above mention technical problems required by clients with best Customer Support.
- Novel Idea
- Ontime Delivery
- Best Prices
- Unique Work
Simulation Projects Workflow

Embedded Projects Workflow
