Operations Research Python we offer sseveral important libraries are highly useful and appropriate to carry out operations research in Python. So stay in contact with matlabprojects.org we offer you novel research help with best thesis ideas and topics along with implementation help. By considering numerous major libraries and their way of application, we provide a concise outline in an explicit manner:
Major Libraries for Operations Research in Python
- NumPy:
- For extensive multi-dimensional matrices and arrays, this library offers assistance.
- To work on these arrays, mathematical functions are provided by this library.
- SciPy:
- In order to support optimization, statistics, combination, and linear algebra, SciPy offers supplementary functionality. It specifically develops on NumPy.
- Pandas:
- Pandas is considered as a robust library for data manipulation and exploration.
- For carrying out complicated data processes and managing datasets, it is more helpful.
- PuLP:
- PuLP is typically written in Python, and is a linear programming modeler.
- To define optimization issues, it offers extensive support. Through various solvers, it assists us to resolve these issues efficiently.
- CVXPY:
- For convex optimization issues, CVXPY is considered as a modeling architecture.
- It has the ability to manage intricate optimization issues and facilitates various types of solvers.
- OR-Tools:
- Particularly for combinatorial optimization, linear programming, and constraint programming, it offers efficient tools. This library is created by Google.
Sample Applications
Linear Programming with PuLP
By means of PuLP, we plan to resolve a linear programming problem in this basic instance:
import pulp
# Define the problem
problem = pulp.LpProblem(“SimpleLP”, pulp.LpMaximize)
# Define decision variables
x = pulp.LpVariable(‘x’, lowBound=0)
y = pulp.LpVariable(‘y’, lowBound=0)
# Objective function
problem += 3 * x + 2 * y, “Objective”
# Constraints
problem += 2 * x + y <= 20
problem += 4 * x – 5 * y >= -10
problem += x + 2 * y <= 15
# Solve the problem
problem.solve()
# Print the results
print(f”Status: {pulp.LpStatus[problem.status]}”)
print(f”x = {pulp.value(x)}”)
print(f”y = {pulp.value(y)}”)
print(f”Objective = {pulp.value(problem.objective)}”)
Integer Programming with PuLP
Focus on resolving an integer programming problem in this instance:
import pulp
# Define the problem
problem = pulp.LpProblem(“SimpleIP”, pulp.LpMaximize)
# Define decision variables (as integers)
x = pulp.LpVariable(‘x’, lowBound=0, cat=’Integer’)
y = pulp.LpVariable(‘y’, lowBound=0, cat=’Integer’)
# Objective function
problem += 3 * x + 2 * y, “Objective”
# Constraints
problem += 2 * x + y <= 20
problem += 4 * x – 5 * y >= -10
problem += x + 2 * y <= 15
# Solve the problem
problem.solve()
# Print the results
print(f”Status: {pulp.LpStatus[problem.status]}”)
print(f”x = {pulp.value(x)}”)
print(f”y = {pulp.value(y)}”)
print(f”Objective = {pulp.value(problem.objective)}”
Convex Optimization with CVXPY
In this instance, a convex optimization problem has to be resolved:
import cvxpy as cp
# Define the problem variables
x = cp.Variable()
y = cp.Variable()
# Define the objective function
objective = cp.Maximize(3 * x + 2 * y)
# Define the constraints
constraints = [2 * x + y <= 20,
4 * x – 5 * y >= -10,
x + 2 * y <= 15,
x >= 0,
y >= 0]
# Formulate the problem
problem = cp.Problem(objective, constraints)
# Solve the problem
problem.solve()
# Print the results
print(f”Status: {problem.status}”)
print(f”x = {x.value}”)
print(f”y = {y.value}”)
print(f”Objective = {problem.value}”)
Initiation Process
It is crucial to install the required libraries to initiate the operations research using Python. Through pip, we can install the relevant libraries:
pip install numpy scipy pandas pulp cvxpy ortools
Operations research python Project Topics
Related to Operations Research, numerous topics and ideas are continuously emerging, which are examined as significant as well as interesting. By emphasizing operations research, we suggest 50 important project topics that can be explored through the use of Python:
- Supply Chain Optimization
- Explanation: To reduce expenses in addition to preserving service ranges, the logistics and distribution network has to be enhanced by creating a model.
- Required Tools: SciPy and PuLP.
- Vehicle Routing Problem (VRP)
- Explanation: For a group of vehicles which supply products to different places, the ideal paths must be identified through developing an algorithm.
- Required Tools: OR-Tools.
- Production Scheduling
- Explanation: To minimize interruptions and enhance effectiveness in a factory, we improve the manufacturing operations by creating a scheduling framework.
- Required Tools: Pandas and PuLP.
- Workforce Scheduling
- Explanation: In addition to reducing labor expenses, plan to align with the requirement by enhancing workers schedules. To accomplish this task, a model has to be developed.
- Required Tools: Pandas and PuLP.
- Portfolio Optimization
- Explanation: As a means to reduce risk and improve profits in an investment portfolio, the allotment of assets should be enhanced through creating a model.
- Required Tools: CVXPY.
- Network Flow Optimization
- Explanation: To enhance throughput or reduce expenses, the flow of resources must be improved across a network.
- Required Tools: PuLP and NetworkX.
- Inventory Management
- Explanation: By stabilizing shortage risks and holding costs, handle inventory ranges in an effective manner by developing a framework.
- Required Tools: Pandas and SciPy.
- Queueing Theory Applications
- Explanation: With the intentions of enhancing the service standard and minimizing wait durations, we improve service facilities (for instance: hospitals, call centers) through creating models.
- Required Tools: NumPy and SciPy.
- Dynamic Pricing
- Explanation: On the basis of demand variations, improve income by applying a dynamic pricing policy.
- Required Tools: CVXPY and Pandas.
- Knapsack Problem
- Explanation: For capacity scheduling and resource allocation, the fluctuations of the knapsack problem have to be resolved.
- Required Tools: SciPy and PuLP.
- Facility Location Problem
- Explanation: To reduce operational and transportation expenses, the ideal places must be identified for amenities (for instance: stores, warehouses).
- Required Tools: OR-Tools and PuLP.
- Cutting Stock Problem
- Explanation: In order to align with requirements and reduce waste, the cutting of raw materials has to be enhanced.
- Required Tools: SciPy and PuLP.
- Traveling Salesman Problem (TSP)
- Explanation: To identify the shortest potential path, an efficient solution should be created for the TSP. For precisely one time, the path must visit every city and revert to the beginning city.
- Required Tools: OR-Tools.
- Production Planning
- Explanation: As a means to align with the requirement at low cost, we enhance the production schedule by developing a model.
- Required Tools: Pandas and PuLP.
- Airline Crew Scheduling
- Explanation: To adhere to rules and reduce expenses, the planning of airline companies has to be improved.
- Required Tools: Pandas and PuLP.
- Healthcare Resource Allocation
- Explanation: At the time of pandemics, healthcare resources (for instance: ventilators, beds) have to be allocated in an effective manner. For that, create a framework.
- Required Tools: PuLP and SciPy.
- Capital Budgeting
- Explanation: In order to improve the net present value (NPV), the allotment of capital investments must be enhanced.
- Required Tools: Pandas and CVXPY.
- Telecommunication Network Design
- Explanation: To assure low latency and effective flow of data, we aim to enhance the telecommunications networks’ model.
- Required Tools: PuLP and NetworkX.
- Revenue Management
- Explanation: In various sectors such as airlines and hotels, plan to enhance income by applying a revenue management framework.
- Required Tools: CVXPY and Pandas.
- Energy Management in Smart Grids
- Explanation: Enhance effectiveness and minimize expenses in smart grids through improving energy usage and distribution.
- Required Tools: Pandas and SciPy.
- Manufacturing Process Optimization
- Explanation: To enhance productivity and minimize waste, different production operations have to be improved. For that, build efficient models.
- Required Tools: Pandas and SciPy.
- Classroom Scheduling
- Explanation: In an academic institution, the planning of rooms and classes should be enhanced for usage improvement.
- Required Tools: Pandas and PuLP.
- Project Scheduling
- Explanation: As a means to align with resource boundaries and time-limits, we improve project plans by developing a model.
- Required Tools: Pandas and PuLP.
- Logistics Network Design
- Explanation: To reduce warehousing and transportation expenses, a logistics network has to be modeled.
- Required Tools: OR-Tools and PuLP.
- Retail Assortment Optimization
- Explanation: In order to provide in a retail store, the choice of products must be improved for efficiency and sales enhancement.
- Required Tools: Pandas and CVXPY.
- Maintenance Scheduling
- Explanation: With the aim of reducing maintenance expenses and interruptions, our project creates a maintenance planning framework.
- Required Tools: Pandas and PuLP.
- Sales Territory Design
- Explanation: To enhance sales and stabilize workloads, the structure of sales areas should be improved.
- Required Tools: PuLP and SciPy.
- Diet Problem
- Explanation: By aligning with nutritional needs at low cost, we develop diet strategies through building a model.
- Required Tools: Pandas and PuLP.
- Portfolio Rebalancing
- Explanation: As a means to preserve optimal risk and return profiles, realign investment portfolios over time by developing an efficient algorithm.
- Required Tools: Pandas and CVXPY.
- Call Center Optimization
- Explanation: To minimize expenses and enhance service ranges, the processes of a call center have to be improved.
- Required Tools: Pandas and SciPy.
- Election Campaign Optimization
- Explanation: The possibility of achieving in election has to be improved by assigning campaign resources in an effective way. For that, create a model.
- Required Tools: PuLP and SciPy.
- Traffic Signal Optimization
- Explanation: In order to enhance traffic flow and minimize congestion, the traffic signal timing has to be improved.
- Required Tools: OR-Tools and PuLP.
- Drone Delivery Optimization
- Explanation: For drone supplies, the plans and paths have to be enhanced by developing a model.
- Required Tools: SciPy and OR-Tools.
- Telecom Network Expansion
- Explanation: To reduce expenses and improve coverage, the growth of telecom networks must be enhanced.
- Required Tools: PuLP and NetworkX.
- Production-Inventory Coordination
- Explanation: With the intention of reducing expenses, we synchronize inventory handling and production through creating a model.
- Required Tools: Pandas and SciPy.
- Pharmaceutical R&D Portfolio Optimization
- Explanation: As a means to increase the anticipated profit in the pharmaceutical sector, the portfolio of research and development projects should be enhanced.
- Required Tools: Pandas and CVXPY.
- Telecom Fraud Detection
- Explanation: In telecommunication networks, focus on identifying and obstructing fraud by applying a model.
- Required Tools: Pandas and SciPy.
- E-commerce Product Recommendation
- Explanation: To improve sales, products have to be recommended to consumers through creating a recommendation framework.
- Required Tools: SciPy and Pandas.
- Inventory Routing Problem
- Explanation: In order to reduce expenses, the routing of vehicles should be enhanced for inventory refilling.
- Required Tools: SciPy and OR-Tools.
- Transportation Problem
- Explanation: Specifically in carrying products from numerous origins to numerous destinations, the expense has to be reduced. For that, we resolve the transportation issue.
- Required Tools: PuLP.
- Employee Shift Scheduling
- Explanation: In addition to reducing labor expenses, focus on aligning with employee needs by developing a shift scheduling framework.
- Required Tools: Pandas and PuLP.
- Sports Tournament Scheduling
- Explanation: To reduce disputes and enhance attendance, the planning of sports tournaments must be improved.
- Required Tools: OR-Tools and PuLP.
- Auction Design and Optimization
- Explanation: As a means to improve social benefit or income, efficient models must be created for auction plans.
- Required Tools: Pandas and CVXPY.
- Resource Allocation in Cloud Computing
- Explanation: In cloud computing platforms, we plan to improve the resource allocation for efficacy enhancement.
- Required Tools: Pandas and SciPy.
- Disaster Response Logistics
- Explanation: At the time of disaster response, the dissemination of resources has to be enhanced by creating a logistics strategy.
- Required Tools: OR-Tools and PuLP.
- Environmental Impact Assessment
- Explanation: The ecological effect of different industrial operations should be evaluated and reduced through developing models.
- Required Tools: Pandas and SciPy.
- Water Distribution Network Optimization
- Explanation: To assure consistent supply and reduce expenses, the process of water distribution networks must be improved.
- Required Tools: PuLP and NetworkX.
- Capacity Planning for Hospitals
- Explanation: In order to manage patient requirements in an effective manner, the hospital range has to be designed and enhanced by creating models.
- Required Tools: Pandas and SciPy.
- Supply Chain Risk Management
- Explanation: Particularly in supply chain processes, we detect and reduce potential risks through developing models.
- Required Tools: Pandas and SciPy.
- Electric Vehicle Charging Station Placement
- Explanation: Consider electric vehicle charging stations and enhance their deployment for cost reduction and coverage improvement.
- Required Tools: PuLP and OR-Tools.
Appropriate for operations research in Python, we recommended several important libraries, along with concise outlines and sample applications. Relevant to operations research, numerous compelling project topics are proposed by us, including brief explanations and required tools.
Subscribe Our Youtube Channel
You can Watch all Subjects Matlab & Simulink latest Innovative Project Results
Our services
We want to support Uncompromise Matlab service for all your Requirements Our Reseachers and Technical team keep update the technology for all subjects ,We assure We Meet out Your Needs.
Our Services
- Matlab Research Paper Help
- Matlab assignment help
- Matlab Project Help
- Matlab Homework Help
- Simulink assignment help
- Simulink Project Help
- Simulink Homework Help
- Matlab Research Paper Help
- NS3 Research Paper Help
- Omnet++ Research Paper Help
Our Benefits
- Customised Matlab Assignments
- Global Assignment Knowledge
- Best Assignment Writers
- Certified Matlab Trainers
- Experienced Matlab Developers
- Over 400k+ Satisfied Students
- Ontime support
- Best Price Guarantee
- Plagiarism Free Work
- Correct Citations
Expert Matlab services just 1-click
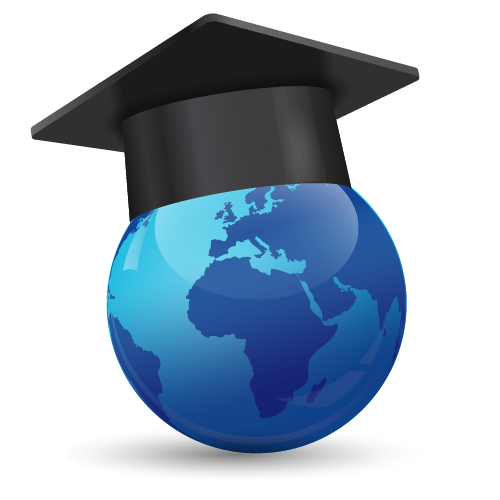
Delivery Materials
Unlimited support we offer you
For better understanding purpose we provide following Materials for all Kind of Research & Assignment & Homework service.
Programs
Designs
Simulations
Results
Graphs
Result snapshot
Video Tutorial
Instructions Profile
Sofware Install Guide
Execution Guidance
Explanations
Implement Plan
Matlab Projects
Matlab projects innovators has laid our steps in all dimension related to math works.Our concern support matlab projects for more than 10 years.Many Research scholars are benefited by our matlab projects service.We are trusted institution who supplies matlab projects for many universities and colleges.
Reasons to choose Matlab Projects .org???
Our Service are widely utilized by Research centers.More than 5000+ Projects & Thesis has been provided by us to Students & Research Scholars. All current mathworks software versions are being updated by us.
Our concern has provided the required solution for all the above mention technical problems required by clients with best Customer Support.
- Novel Idea
- Ontime Delivery
- Best Prices
- Unique Work
Simulation Projects Workflow

Embedded Projects Workflow
